Users Online
· Guests Online: 32
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
Forum Threads
Newest Threads
No Threads created
Hottest Threads
No Threads created
Latest Articles
Articles Hierarchy
031 Java Android Program to Demonstrate a Simple to do List Application
Java Android Program to Demonstrate a Simple to do List Application
This Android Java Program lets you create an Activity to create a Simple to do List Application.
Here is source code of the Program to create an Activity to create a Simple to do List Application. The program is successfully compiled and run on a Windows system using Eclipse Ide. The program output is also shown below.
Main Activity
package com.example.to_do_list;
import java.util.ArrayList;
import android.R.string;
import android.os.Bundle;
import android.app.Activity;
import android.view.KeyEvent;
import android.view.Menu;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.EditText;
import android.widget.ListView;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ListView lv = (ListView) findViewById(R.id.ListView);
final EditText text = (EditText) findViewById(R.id.edittext);
final ArrayList do_list = new ArrayList();
final ArrayAdapter adapter;
adapter = new ArrayAdapter(this,
android.R.layout.simple_list_item_1, do_list);
lv.setAdapter(adapter);
text.setOnKeyListener(new View.OnKeyListener() {
@Override
public boolean onKey(View v, int keyCode, KeyEvent event) {
// TODO Auto-generated method stub
if (event.getAction() == KeyEvent.ACTION_DOWN) {
if ((keyCode == KeyEvent.KEYCODE_DPAD_CENTER)
|| (keyCode == KeyEvent.KEYCODE_ENTER)) {
do_list.add(0, text.getText().toString());
adapter.notifyDataSetChanged();
text.setText("");
return true;
}
}
return false;
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
DolistitemView
package com.example.to_do_list;
import android.content.Context;
import android.content.res.Resources;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.util.AttributeSet;
import android.widget.TextView;
public class dolistitemview extends TextView {
private Paint marginPaint;
private Paint linePaint;
private int paperColor;
private float margin;
public dolistitemview(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
// TODO Auto-generated constructor stub
init();
}
private void init() {
Resources myResources = getResources();
marginPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
marginPaint.setColor(myResources.getColor(R.color.notepad_margin));
linePaint = new Paint(Paint.ANTI_ALIAS_FLAG);
linePaint.setColor(myResources.getColor(R.color.notepad_lines));
paperColor = myResources.getColor(R.color.notepad_paper);
margin = myResources.getDimension(R.dimen.notepad_margin);
}
@Override
public void onDraw(Canvas canvas) {
// Use the base TextView to render the text.
canvas.drawColor(paperColor);
//drawing lines
canvas.drawLine(0, 0, 0, getMeasuredHeight(), linePaint);
canvas.drawLine(0, getMeasuredHeight(), getMeasuredWidth(),
getMeasuredHeight(), linePaint);
//the margin line
canvas.drawLine(margin, 0, margin, getMeasuredHeight(), marginPaint);
canvas.save();
canvas.translate(margin, 0);
super.onDraw(canvas);
canvas.restore();
}
}
Xml
Main
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<EditText
android:id="@+id/edittext"
android:layout_width="fill_parent"
android:layout_height="wrap_content" />
<ListView
android:id="@+id/ListView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
Dolistitem Xml
<?xml version="1.0" encoding="utf-8"?>
<com.example.to_do_list.dolistitemview xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fadingEdge="vertical"
android:padding="10dp"
android:scrollbars="vertical"
android:textColor="@color/notepad_text" />
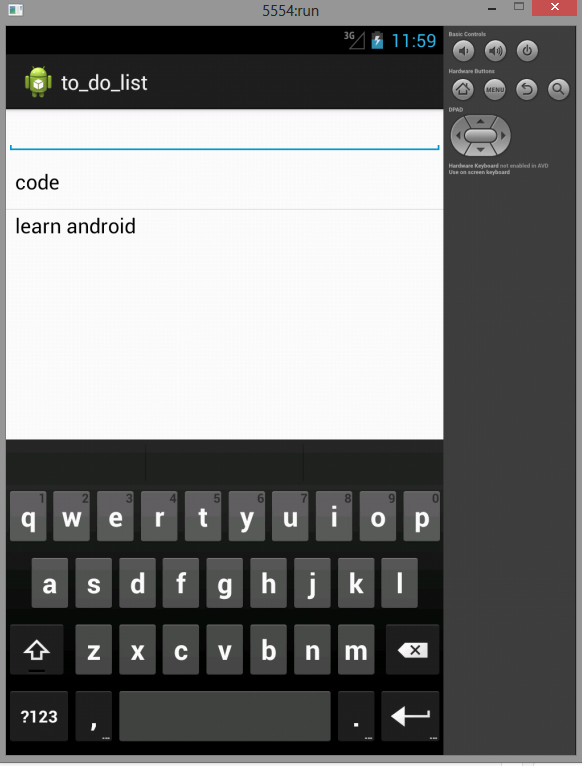
This Android Java Program lets you create an Activity to create a Simple to do List Application.
Here is source code of the Program to create an Activity to create a Simple to do List Application. The program is successfully compiled and run on a Windows system using Eclipse Ide. The program output is also shown below.
Main Activity
package com.example.to_do_list;
import java.util.ArrayList;
import android.R.string;
import android.os.Bundle;
import android.app.Activity;
import android.view.KeyEvent;
import android.view.Menu;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.EditText;
import android.widget.ListView;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ListView lv = (ListView) findViewById(R.id.ListView);
final EditText text = (EditText) findViewById(R.id.edittext);
final ArrayList
final ArrayAdapter
adapter = new ArrayAdapter
android.R.layout.simple_list_item_1, do_list);
lv.setAdapter(adapter);
text.setOnKeyListener(new View.OnKeyListener() {
@Override
public boolean onKey(View v, int keyCode, KeyEvent event) {
// TODO Auto-generated method stub
if (event.getAction() == KeyEvent.ACTION_DOWN) {
if ((keyCode == KeyEvent.KEYCODE_DPAD_CENTER)
|| (keyCode == KeyEvent.KEYCODE_ENTER)) {
do_list.add(0, text.getText().toString());
adapter.notifyDataSetChanged();
text.setText("");
return true;
}
}
return false;
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
DolistitemView
package com.example.to_do_list;
import android.content.Context;
import android.content.res.Resources;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.util.AttributeSet;
import android.widget.TextView;
public class dolistitemview extends TextView {
private Paint marginPaint;
private Paint linePaint;
private int paperColor;
private float margin;
public dolistitemview(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
// TODO Auto-generated constructor stub
init();
}
private void init() {
Resources myResources = getResources();
marginPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
marginPaint.setColor(myResources.getColor(R.color.notepad_margin));
linePaint = new Paint(Paint.ANTI_ALIAS_FLAG);
linePaint.setColor(myResources.getColor(R.color.notepad_lines));
paperColor = myResources.getColor(R.color.notepad_paper);
margin = myResources.getDimension(R.dimen.notepad_margin);
}
@Override
public void onDraw(Canvas canvas) {
// Use the base TextView to render the text.
canvas.drawColor(paperColor);
//drawing lines
canvas.drawLine(0, 0, 0, getMeasuredHeight(), linePaint);
canvas.drawLine(0, getMeasuredHeight(), getMeasuredWidth(),
getMeasuredHeight(), linePaint);
//the margin line
canvas.drawLine(margin, 0, margin, getMeasuredHeight(), marginPaint);
canvas.save();
canvas.translate(margin, 0);
super.onDraw(canvas);
canvas.restore();
}
}
Xml
Main
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<EditText
android:id="@+id/edittext"
android:layout_width="fill_parent"
android:layout_height="wrap_content" />
<ListView
android:id="@+id/ListView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
Dolistitem Xml
<?xml version="1.0" encoding="utf-8"?>
<com.example.to_do_list.dolistitemview xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fadingEdge="vertical"
android:padding="10dp"
android:scrollbars="vertical"
android:textColor="@color/notepad_text" />
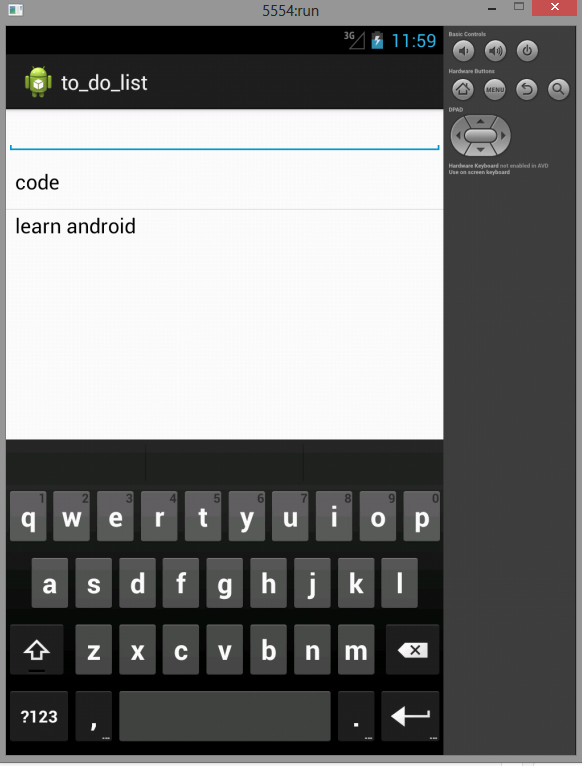
Comments
No Comments have been Posted.
Post Comment
Please Login to Post a Comment.