Users Online
· Guests Online: 32
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
Forum Threads
Newest Threads
No Threads created
Hottest Threads
No Threads created
Latest Articles
Articles Hierarchy
016 Java Android Program for Dividing our Activity into Fully Encapsulated Reusable Components using Fragement
Java Android Program for Dividing our Activity into Fully Encapsulated Reusable Components using Fragement
This Android Java Program lets you create an Activity using Fragements.
Here is source code of the Program to create an Activity using Fragements. The program is successfully compiled and run on a Windows system using Eclipse Ide. The program output is also shown below.
A Fragments is an independent component which can be connected to an activity. A Fragment typically defines a part of a user interface but it is possible to define headless Fragments, i.e. without user interface.
Fragments can be dynamically or statically added to a layout. A Fragment encapsulate functionality so that it is easier to reuse within activity and layouts.
A Fragment component runs in the context of an activity but it has its own lifecycle and their own user interface.
Main
package com.example.create_fragment;
import android.os.Bundle;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.view.Menu;
//creating two fragments in an activity to show the current time in milli secs.
@SuppressLint("NewApi")
public class MainActivity extends Activity implements fragment2.Listener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public void onItemSelected(String str) {
// TODO Auto-generated method stub
fragment1 fragment = (fragment1) getFragmentManager().findFragmentById(
R.id.Fragment1);
if (fragment != null && fragment.isInLayout()) {
fragment.setText(str);
}
}
}
Fragement1
package com.example.create_fragment;
import android.annotation.SuppressLint;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
@SuppressLint("NewApi")
public class fragment1 extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment1, container, false);
return view;
}
public void setText(String item) {
TextView view = (TextView) getView().findViewById(R.id.textView1);
view.setText(item);
}
}
Fragement2
package com.example.create_fragment;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Fragment;
import android.os.Bundle;
import android.os.SystemClock;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
@SuppressLint("NewApi")
public class fragment2 extends Fragment {
private Listener listener;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment2, container, false);
Button button = (Button) view.findViewById(R.id.button1);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
updateDetail();
}
});
return view;
}
public interface Listener {
public void onItemSelected(String link);
}
@Override
public void onAttach(Activity activity) {
super.onAttach(activity);
if (activity instanceof Listener) {
listener = (Listener) activity;
} else {
throw new ClassCastException(activity.toString()
+ " must implemenet fragement2.listener");
}
}
public void updateDetail() {
String newTime = String.valueOf(System.currentTimeMillis());
listener.onItemSelected(newTime);
}
@Override
public void onDetach() {
// TODO Auto-generated method stub
super.onDetach();
listener = null;
}
}
Xml
Main Xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity"
android:background="@android:color/background_dark" >
<fragment
android:id="@+id/Fragment1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="?android:attr/actionBarSize"
class="com.example.create_fragment.fragment1" >
</fragment>
<fragment
android:id="@+id/Fragment2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
class="com.example.create_fragment.fragment2" >
</fragment>
</RelativeLayout>
Fragement1
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/darker_gray"
android:orientation="vertical" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal|center_vertical"
android:layout_marginTop="20dip"
android:text="Large Text"
android:textAppearance="?android:attr/textAppearanceLarge"
android:textSize="30dp" />
</LinearLayout>
Fragement2
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Press to update" />
</LinearLayout>
AndroidManifest
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.create_fragment"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="17" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.example.create_fragment.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
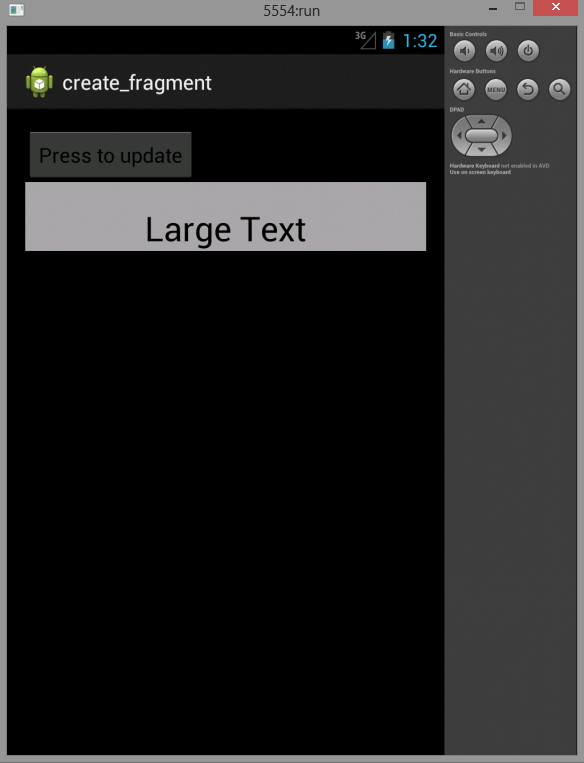
This Android Java Program lets you create an Activity using Fragements.
Here is source code of the Program to create an Activity using Fragements. The program is successfully compiled and run on a Windows system using Eclipse Ide. The program output is also shown below.
A Fragments is an independent component which can be connected to an activity. A Fragment typically defines a part of a user interface but it is possible to define headless Fragments, i.e. without user interface.
Fragments can be dynamically or statically added to a layout. A Fragment encapsulate functionality so that it is easier to reuse within activity and layouts.
A Fragment component runs in the context of an activity but it has its own lifecycle and their own user interface.
Main
package com.example.create_fragment;
import android.os.Bundle;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.view.Menu;
//creating two fragments in an activity to show the current time in milli secs.
@SuppressLint("NewApi")
public class MainActivity extends Activity implements fragment2.Listener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public void onItemSelected(String str) {
// TODO Auto-generated method stub
fragment1 fragment = (fragment1) getFragmentManager().findFragmentById(
R.id.Fragment1);
if (fragment != null && fragment.isInLayout()) {
fragment.setText(str);
}
}
}
Fragement1
package com.example.create_fragment;
import android.annotation.SuppressLint;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
@SuppressLint("NewApi")
public class fragment1 extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment1, container, false);
return view;
}
public void setText(String item) {
TextView view = (TextView) getView().findViewById(R.id.textView1);
view.setText(item);
}
}
Fragement2
package com.example.create_fragment;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.app.Fragment;
import android.os.Bundle;
import android.os.SystemClock;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
@SuppressLint("NewApi")
public class fragment2 extends Fragment {
private Listener listener;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment2, container, false);
Button button = (Button) view.findViewById(R.id.button1);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
updateDetail();
}
});
return view;
}
public interface Listener {
public void onItemSelected(String link);
}
@Override
public void onAttach(Activity activity) {
super.onAttach(activity);
if (activity instanceof Listener) {
listener = (Listener) activity;
} else {
throw new ClassCastException(activity.toString()
+ " must implemenet fragement2.listener");
}
}
public void updateDetail() {
String newTime = String.valueOf(System.currentTimeMillis());
listener.onItemSelected(newTime);
}
@Override
public void onDetach() {
// TODO Auto-generated method stub
super.onDetach();
listener = null;
}
}
Xml
Main Xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity"
android:background="@android:color/background_dark" >
<fragment
android:id="@+id/Fragment1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="?android:attr/actionBarSize"
class="com.example.create_fragment.fragment1" >
</fragment>
<fragment
android:id="@+id/Fragment2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
class="com.example.create_fragment.fragment2" >
</fragment>
</RelativeLayout>
Fragement1
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/darker_gray"
android:orientation="vertical" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal|center_vertical"
android:layout_marginTop="20dip"
android:text="Large Text"
android:textAppearance="?android:attr/textAppearanceLarge"
android:textSize="30dp" />
</LinearLayout>
Fragement2
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Press to update" />
</LinearLayout>
AndroidManifest
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.create_fragment"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="17" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.example.create_fragment.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
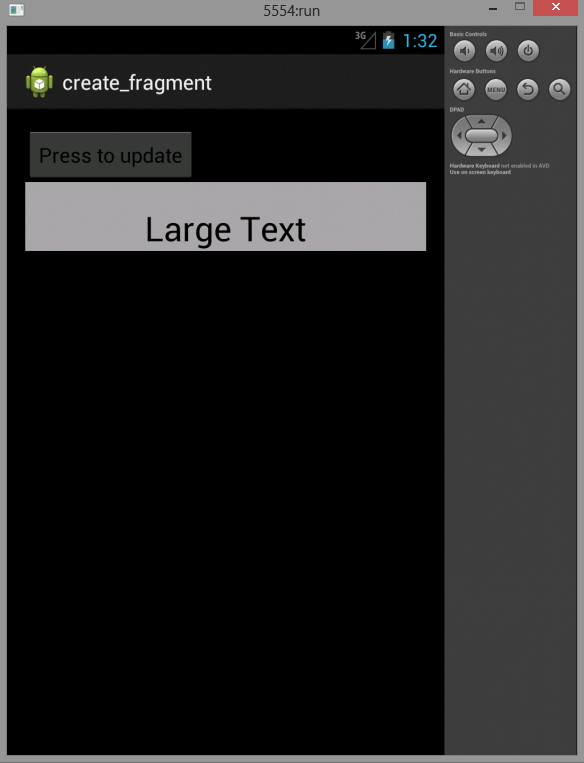
Comments
No Comments have been Posted.
Post Comment
Please Login to Post a Comment.