Users Online
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
Forum Threads
Latest Articles
Articles Hierarchy
A Guide to Service Workers in React.js
A Guide to Service Workers in React.js
A Guide to Service Workers in React.js
If you’ve ever tried to start your own app (create-react-app
) in React for the first time, you may have noticed that a service worker class is invoked by default. If you’re anything like me, you may be wondering: what does this service worker do?
I am going to take you through what a service worker is, why it was created, and how you can use it in your apps.
What is a service worker?
A service worker is a script that the client (your browser) runs in the background separate from the web page. Operating in the background separate from the web page enables you to use features that don’t actually require a web page or user interaction to trigger — like a push notification or background sync.
Why is this API such an exciting development? Because it allows you to support offline experiences, giving engineers and developers end-to-end control over the user’s interactions with the app. A service worker enables you to run JavaScript before a page even exists, makes your site faster, and allows you to display content even if there is no internet connection.
Woah!Key Traits and Properties
As you can tell, service workers are extremely important in creating a Progressive Web App (learn more about PWA’s here). Before I dive into how to implement a service worker, here are some key traits and properties that it has:
- Runs in its own global script context
- Is not directly tied to any particular page
- Cannot access the DOM
- Is event-driven (it’s terminated when it’s not in use and run again when needed)
- Is HTTPS only
There are some pretty interesting things to note about a service worker. Since it can’t access the DOM directly, it needs a workaround to communicate with the pages it controls. It does this by responding to messages sent via the postMessage
interface (through a MessagePort
), allowing those pages it’s controlling to manipulate the DOM as directed. Additionally, being event-driven, you can’t rely on state in a service worker’s onfetch
and onmessage
handlers to persist any data. According to the React documentation, if there is information that you need to persist and reuse across restarts, service workers have access to the IndexedDB API.
It’s clear that service workers are complicated yet powerful tools that we have at our disposal. What’s even better? React sets us up for success with implementing them just by running create-react-app
. In this next section, I’ll walk you through how to set up a service worker with your React app.
Please note that installing and setting up service workers in general without React is a much lengthier process. In this blog, I’ll just be taking you through how to set up a service worker in React, but you can read the full documentation on how to implement service workers anywhere here.
How do I use a service worker?
After creating a new React app, you’ll see the following file structure created for you:

By default, the React build process will generate a serviceWorker.js
file in your src
folder. However, the default for the service worker is unregistered, meaning that it won’t yet be able to take control of your web app.
So, we’ll need to first opt-in to the offline-first behavior. In your src/index.js
, you’ll find the following code:

As it states, switching serviceWorker.unregister()
to serviceWorker.register()
will allow you to opt in to using the service worker.
Without React’s handy provided code, you’d have to code the entire service worker lifecycle. Here’s a brief overview on what that looks like via Google documentation:
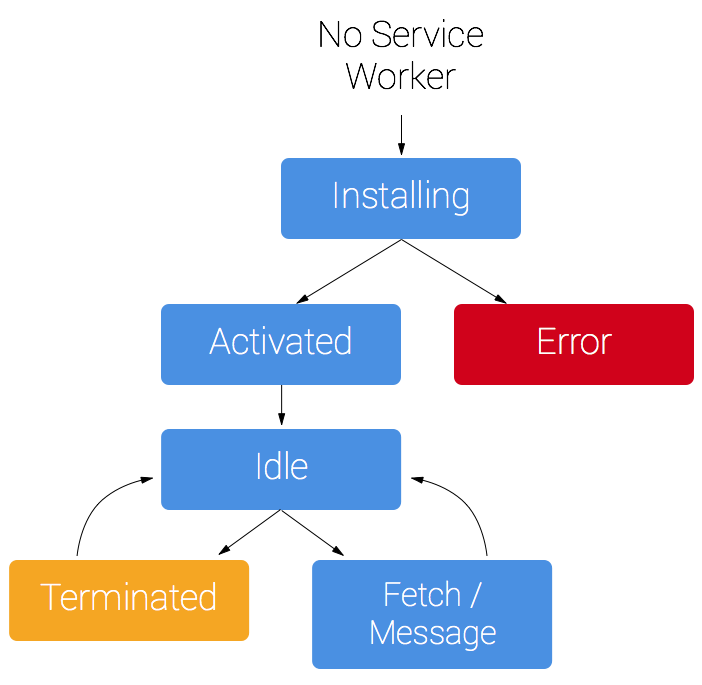
So, you’d have to manually code the register → install → cache & return requests → so on and so forth process.
Luckily, we have React! If you pop into your src/serviceWorker.js
file, you’ll see a bunch of pre-loaded code with comments:





Cool! Setting serviceWorker.register()
runs all the above code, and now you’re ready to hit the ground running with your service worker.
The benefits of opting-in to using the service worker are great, including creating faster and more reliable web apps and providing a more engaging mobile experience. However, just like most functionalities, there are some things to take into account if you decide to opt-in to service worker registration. You can learn about those in the official React.js documentation here.
So, the next time you get a push notification when your friend likes your Instagram or you start receiving ads for a local business the minute you set foot in that store’s town — you have service helpers to thank for that!