Users Online
· Guests Online: 4
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
Forum Threads
Newest Threads
No Threads created
Hottest Threads
No Threads created
Latest Articles
Articles Hierarchy
160 Java Android Program to Demonstrate Parsing a Json Object
Here is source code of the Program to Demonstrate Parsing a Json Object in Android. The program is successfully compiled and run on a Windows system using Eclipse Ide. The program output is also shown below.
Main Activity
package com.example.json_app;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
// URL to get JSON Array
private static String url = "http://evaluate.rome2rio.com/api/1.2/json/Search?key=fWAL2WvM&oName=Dwarka+Delhi&dName=Hauz+Khas+Delhi";
JSONObject json = null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Creating new JSON Parser
final JSONParser jParser = new JSONParser();
// Getting JSON from URL
final TextView text = (TextView) findViewById(R.id.text);
new AsyncTask() {
@Override
protected Void doInBackground(Void... params) {
json = jParser.getJSONFromUrl(url);
return null;
}
@Override
protected void onPostExecute(Void result) {
super.onPostExecute(result);
double tot_price = 0;
try {
JSONArray routes = json.getJSONArray("routes");
for (int i = 0; i < routes.length(); i++) {
JSONObject obj = routes.getJSONObject(i);
String name = obj.getString("name");
if (name.contains("Metro")) {
System.out.println(obj.get("distance"));
// System.out.println(obj.get("segments"));
JSONArray segemnts = obj.getJSONArray("segments");
for (int j = 0; j < segemnts.length(); j++) {
JSONObject seg = segemnts.getJSONObject(j);
String name1 = seg.getString("kind");
if (name1.equals("train")) {
String name2 = seg.getString("vehicle");
if (name2.equals("Metro")) {
System.out.println(seg
.getString("sPos"));
JSONArray iten = seg
.getJSONArray("itineraries");
for (int k = 0; k < iten.length(); k++) {
JSONObject it = iten
.getJSONObject(k);
for (int h = 0; h < it.length(); h++) {
JSONArray legs = it
.getJSONArray("legs");
JSONObject leg = legs
.getJSONObject(h);
JSONArray hops = leg
.getJSONArray("hops");
for (int o = 0; o < hops
.length(); o++) {
JSONObject hop = hops
.getJSONObject(o);
System.out
.println(hop
.getString("sName")
+ " "
+ hop.getString("tName"));
JSONObject iprice = hop
.getJSONObject("indicativePrice");
System.out
.println(iprice
.getString("nativePrice"));
tot_price += Double
.parseDouble(iprice
.getString("nativePrice"));
}
}
}
}
}
}
}
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
text.setText("Total Price :" + Double.toString(tot_price));
}
}.execute();
}
}
Json Parser
package com.example.json_app;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONException;
import org.json.JSONObject;
import android.util.Log;
public class JSONParser {
static InputStream is = null;
static JSONObject jObj = null;
static String json = "";
// constructor
public JSONParser() {
}
public JSONObject getJSONFromUrl(String url) {
// Making HTTP request
try {
// defaultHttpClient
DefaultHttpClient httpClient = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
HttpResponse httpResponse = httpClient.execute(httpPost);
HttpEntity httpEntity = httpResponse.getEntity();
is = httpEntity.getContent();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(
is, "iso-8859-1"), 8);
StringBuilder sb = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
Log.i("Tag",line);
sb.append(line + "\n");
}
is.close();
json = sb.toString();
} catch (Exception e) {
Log.e("Buffer Error", "Error converting result " + e.toString());
}
// try parse the string to a JSON object
try {
jObj = new JSONObject(json);
} catch (JSONException e) {
Log.e("JSON Parser", "Error parsing data " + e.toString());
}
// return JSON String
return jObj;
}
}
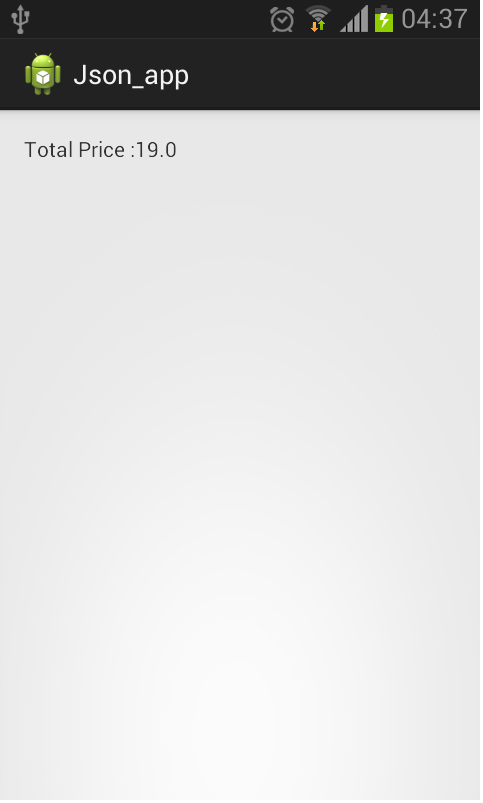
Main Activity
package com.example.json_app;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
// URL to get JSON Array
private static String url = "http://evaluate.rome2rio.com/api/1.2/json/Search?key=fWAL2WvM&oName=Dwarka+Delhi&dName=Hauz+Khas+Delhi";
JSONObject json = null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Creating new JSON Parser
final JSONParser jParser = new JSONParser();
// Getting JSON from URL
final TextView text = (TextView) findViewById(R.id.text);
new AsyncTask
@Override
protected Void doInBackground(Void... params) {
json = jParser.getJSONFromUrl(url);
return null;
}
@Override
protected void onPostExecute(Void result) {
super.onPostExecute(result);
double tot_price = 0;
try {
JSONArray routes = json.getJSONArray("routes");
for (int i = 0; i < routes.length(); i++) {
JSONObject obj = routes.getJSONObject(i);
String name = obj.getString("name");
if (name.contains("Metro")) {
System.out.println(obj.get("distance"));
// System.out.println(obj.get("segments"));
JSONArray segemnts = obj.getJSONArray("segments");
for (int j = 0; j < segemnts.length(); j++) {
JSONObject seg = segemnts.getJSONObject(j);
String name1 = seg.getString("kind");
if (name1.equals("train")) {
String name2 = seg.getString("vehicle");
if (name2.equals("Metro")) {
System.out.println(seg
.getString("sPos"));
JSONArray iten = seg
.getJSONArray("itineraries");
for (int k = 0; k < iten.length(); k++) {
JSONObject it = iten
.getJSONObject(k);
for (int h = 0; h < it.length(); h++) {
JSONArray legs = it
.getJSONArray("legs");
JSONObject leg = legs
.getJSONObject(h);
JSONArray hops = leg
.getJSONArray("hops");
for (int o = 0; o < hops
.length(); o++) {
JSONObject hop = hops
.getJSONObject(o);
System.out
.println(hop
.getString("sName")
+ " "
+ hop.getString("tName"));
JSONObject iprice = hop
.getJSONObject("indicativePrice");
System.out
.println(iprice
.getString("nativePrice"));
tot_price += Double
.parseDouble(iprice
.getString("nativePrice"));
}
}
}
}
}
}
}
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
text.setText("Total Price :" + Double.toString(tot_price));
}
}.execute();
}
}
Json Parser
package com.example.json_app;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONException;
import org.json.JSONObject;
import android.util.Log;
public class JSONParser {
static InputStream is = null;
static JSONObject jObj = null;
static String json = "";
// constructor
public JSONParser() {
}
public JSONObject getJSONFromUrl(String url) {
// Making HTTP request
try {
// defaultHttpClient
DefaultHttpClient httpClient = new DefaultHttpClient();
HttpPost httpPost = new HttpPost(url);
HttpResponse httpResponse = httpClient.execute(httpPost);
HttpEntity httpEntity = httpResponse.getEntity();
is = httpEntity.getContent();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(
is, "iso-8859-1"), 8);
StringBuilder sb = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
Log.i("Tag",line);
sb.append(line + "\n");
}
is.close();
json = sb.toString();
} catch (Exception e) {
Log.e("Buffer Error", "Error converting result " + e.toString());
}
// try parse the string to a JSON object
try {
jObj = new JSONObject(json);
} catch (JSONException e) {
Log.e("JSON Parser", "Error parsing data " + e.toString());
}
// return JSON String
return jObj;
}
}
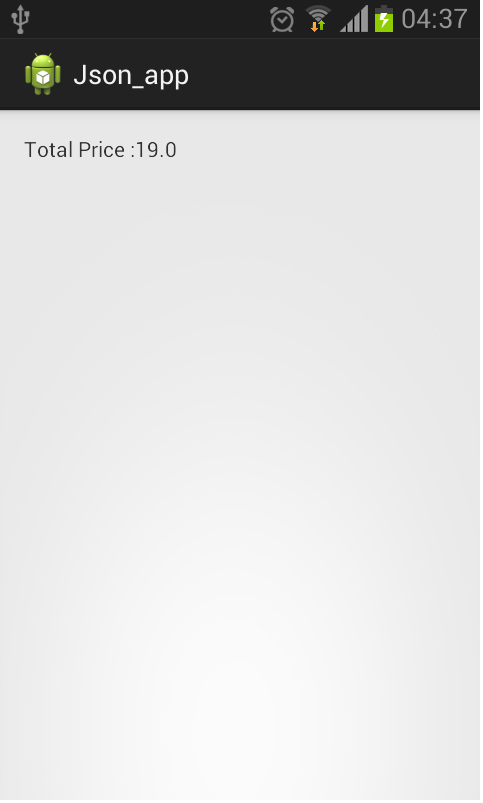
Comments
No Comments have been Posted.
Post Comment
Please Login to Post a Comment.