Users Online
· Guests Online: 89
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
· Members Online: 0
· Total Members: 188
· Newest Member: meenachowdary055
Forum Threads
Newest Threads
No Threads created
Hottest Threads
No Threads created
Latest Articles
Articles Hierarchy
159 Java Android Program to Demonstrate Progress Dialog with Spinning Wheel in Android
Here is source code of the Program to Demonstrate Progress Dialog with Spinning Wheel in Android. The program is successfully compiled and run on a Windows system using Eclipse Ide. The program output is also shown below.
In order to display Progress Dialog box with a ring simply call
ProgressDialog progressdialog = ProgressDialog.show(
MainActivity.this, "Please wait",
"Loading please wait..", true);
The rest of the code is given below.
MainActivity.java
package com.example.progressdialogspinnigwheel;
import android.app.Activity;
import android.app.ProgressDialog;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button = (Button) findViewById(R.id.button1);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
final ProgressDialog progressdialog = ProgressDialog.show(
MainActivity.this, "Please wait",
"Loading please wait..", true);
progressdialog.setCancelable(true);
new Thread(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
try {
// put the thread to sleep for 2 seconds
Thread.sleep(2000);
} catch (Exception e) {
}
progressdialog.dismiss();
}
}).start();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
Activity_Main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/textView1"
android:layout_alignRight="@+id/textView1"
android:layout_below="@+id/textView1"
android:layout_marginTop="80dp"
android:text="Launch" />
</RelativeLayout>
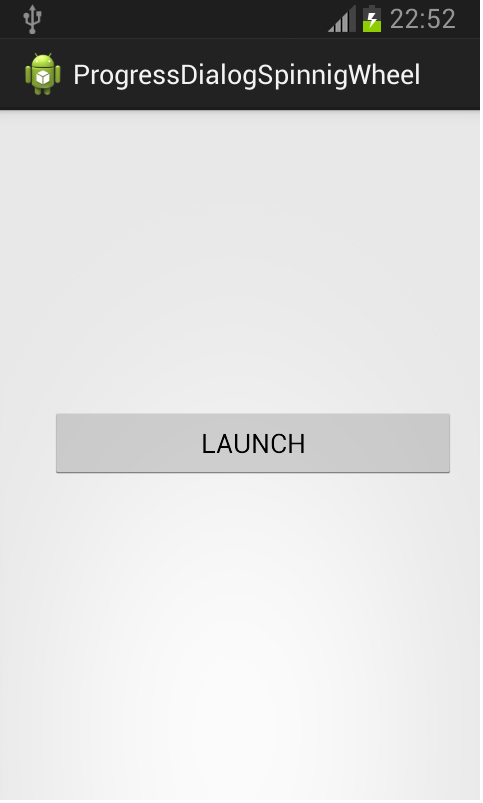
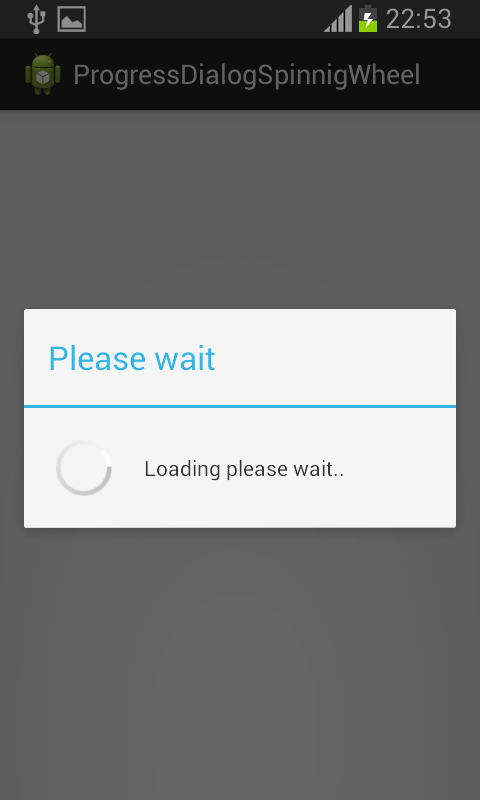
In order to display Progress Dialog box with a ring simply call
ProgressDialog progressdialog = ProgressDialog.show(
MainActivity.this, "Please wait",
"Loading please wait..", true);
The rest of the code is given below.
MainActivity.java
package com.example.progressdialogspinnigwheel;
import android.app.Activity;
import android.app.ProgressDialog;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button = (Button) findViewById(R.id.button1);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
final ProgressDialog progressdialog = ProgressDialog.show(
MainActivity.this, "Please wait",
"Loading please wait..", true);
progressdialog.setCancelable(true);
new Thread(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
try {
// put the thread to sleep for 2 seconds
Thread.sleep(2000);
} catch (Exception e) {
}
progressdialog.dismiss();
}
}).start();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
Activity_Main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/textView1"
android:layout_alignRight="@+id/textView1"
android:layout_below="@+id/textView1"
android:layout_marginTop="80dp"
android:text="Launch" />
</RelativeLayout>
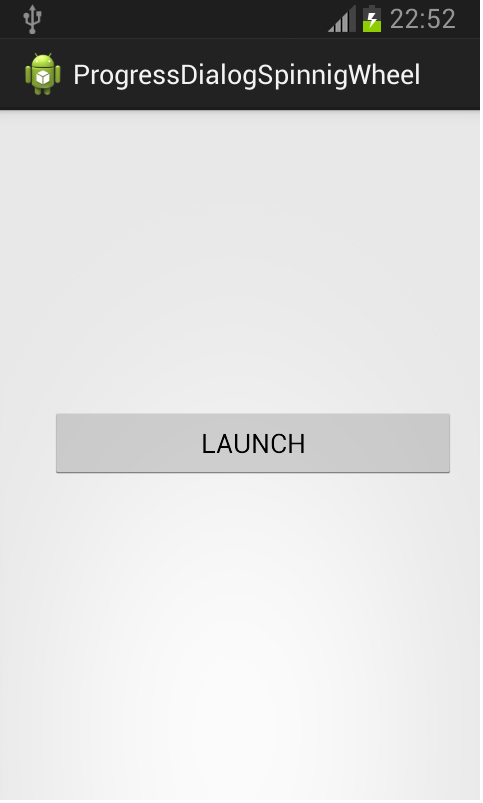
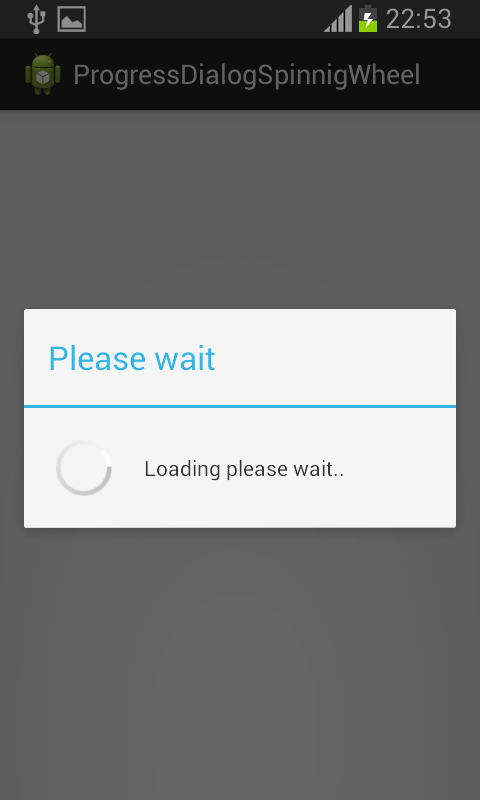
Comments
No Comments have been Posted.
Post Comment
Please Login to Post a Comment.