#C PROGRAMMING LANGUAGE TUTORIALS
Posted by Superadmin on December 25 2020 03:14:31
C PROGRAMMING LANGUAGE TUTORIALS
Introduction - C Programming
1. What is C?
-
C is a programming language developed at AT & T’s Bell Laboratories of USA in 1972 by Dennis Ritchie.
-
Any programming Language can be divided in to two categories.
- Problem oriented (High level language)
- Machine oriented (Low level language)
But C is considered as a Middle level Language
.
-
C is modular
, portable
, reusable
.
2. Feature of C Program
- Structured language
- It has the ability to divide and hide all the information and instruction.
- Code can be partitioned in C using functions or code block.
- C is a well structured language compare to other.
- General purpose language
- Make it ideal language for system programming.
- It can also be used for business and scientific application.
- ANSI established a standard for c in 1983.
- The ability of c is to manipulate bits,byte and addresses.
- It is adopted in later 1990.
- Portability
- Portability is the ability to port or use the software written .
- One computer C program can be reused.
- By modification or no modification.
- Code Re-usability & Ability to customize and extend
- A programmer can easily create his own function
- It can can be used repeatedly in different application
- C program basically collection of function
- The function are supported by 'c' library
- Function can be added to 'c' library continuously
- Limited Number of Key Word
- There are only 32 keywords in 'C'
- 27 keywords are given by ritchie
- 5 is added by ANSI
- The strength of 'C' is lies in its in-built function
- Unix system provides as large number of C function
- Some function are used in operation .
- Other are for specialized in their application
3. C program structure
pre-processor directives
global declarations
main()
{
local variable deceleration
statement sequences
function invoking
}
4. C Keywords
Keywords are the words whose meaning has already been explained to the C compiler. There are only 32 keywords available in C. The keywords are also called ‘Reserved words’.
auto double int struct
break else long switch
case enum register typedef
char extern return union
const float short unsigned
continue for signed void
default goto sizeof volatile
do if static while
5. C Character Set
A character denotes any alphabet, digit or special symbol used to represent information. Following are the valid alphabets, numbers and special symbols allowed in C.
Alphabets
- A, B, ….., Y, Z a, b, ……, y, z
Digits
- 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
Special symbols
- ~ ‘ ! @ # % ^ & * ( ) _ - + = | \ { }
[ ] : ; " ' < > , . ? /
6. Rules for Writing, Compiling and Executing the C program
- C is case sensitive means variable named "COUNTER" is different from a variable named "counter".
- All keywords are lowercased.
- Keywords cannot be used for any other purpose (like variable names).
- Every C statement must end with a
;
. Thus ;acts as a statement terminator.
- First character must be an alphabet or underscore, no special symbol other than an underscore, no commas or blank spaces are allowed with in a variable, constant or keyword.
- Blank spaces may be inserted between two words to improve the readability of the statement. However, no blank spaces are allowed within a variable, constant or keyword.
- Variable must be declared before it is used in the program.
- File should be have the extension .c
- Program need to be compiled before execution.
7. Data types & Placeholders
- C has 5 basic built-in data types.
- Data type defines a set of values that a variable can store along with a set of operations that can be performed on it.
- A variable takes different values at different times.
- General form for declaring a variable is:
type name;
- An example for using variables comes below:
#include<stdio.h>
main()
{
int sum;
sum=12;
sum=sum+5;
printf("Sum is %d",sum);
}
printf
function will print the following:
Sum is 17
In fact %d
is the placeholder for integer variable value that its name comes after double quotes.
- Common data types are:
int
- integer
char
- character
long
- long integer
float
- float number
double
- long float
- Other placeholders are:
Placeholders Format
%c Character
%d Signed decimal integer
%i Signed decimal integer
%e Scientific notation[e]
%E Scientific notation[E]
%f Decimal floating point
%o unsigned octal
%s String of character
%u unsigned decimal integer
%x unsigned Hexadecimal (lower)
%X unsigned Hexadecimal (upper)
%p dispaly a pointer
%% print a %
8. Control characters (Escape sequences)
Certain non printing characters as well as the backslash () and the apostrophe('), can be expressed in terms of escape sequence.
\a
- Bell
\n
- New line
\r
- Carriage return
\b
- Backspace
\f
- Formfeed
\t
- Horizontal tab
\"
- Quotation mark
\v
- Vertical tab
\'
- Apostrophe
\\
- Backslash
\?
- Question mark
\0
- Null
scanf
function used to receiving input from keyboard.
General form of scanf function is :
scanf("Format string",&variable,&variable,...);
Format string
contains placeholders for variables that we intend to receive from keyboard. A &
sign comes before each variable name that comes in variable listing. Character strings are exceptions from this rule. They will not come with this sign before them.
Note: You are not allowed to insert any additional characters in format string other than placeholders and some special characters. Entering even a space or other undesired character will cause your program to work incorrectly and the results will be unexpected. So make sure you just insert placeholder characters in scanf format string. The following example receives multiple variables from keyboard.
float a;
int n;
scanf("%d%f",&n,&a);
Pay attention that scanf function has no error checking capabilities built in it. Programmer is responsible for validating input data (type, range etc.) and preventing errors
Expression & Operators Precedence - C Programming
Following table summaries the rules for precedence and associativity of all operators, including those that we have not yet discussed. Operators on the same row have the same precedence; rows are in order of decreasing precedence, so, for example, *, /, and % all have the same precedence, which is higher than that of binary + and -. The operator () refers to function call. The operators -> and . are used to access members of structures;
DESCRIPTION | OPERATORS | ASSOCIATIVITY |
Function Expression |
() |
Left to Right |
Array Expression |
[] |
Left to Right |
Structure Operator |
-> |
Left to Right |
Structure Operator |
. |
Left to Right |
Unary minus |
- |
Right to Left |
Increment/Decrement |
++, -- |
Right to Left |
One’s compliment |
~ |
Right to Left |
Negation |
! |
Right to Left |
Address of |
& |
Right to Left |
Value of address |
`*` |
Right to Left |
Type cast |
(type) |
Right to Left |
Size in bytes |
sizeof |
Right to Left |
Multiplication |
`*` |
Left to Right |
Division |
/ |
Left to Right |
Modulus |
% |
Left to Right |
Addition |
+ |
Left to Right |
Subtraction |
- |
Left to Right |
Left shift |
<< |
Left to Right |
Right shift |
>> |
Left to Right |
Less than |
< |
Left to Right |
Less than or equal to |
<= |
Left to Right |
Greater than |
> |
Left to Right |
Greater than or equal to |
>= |
Left to Right |
Equal to |
== |
Left to Right |
Not equal to |
!= |
Left to Right |
Bitwise AND |
& |
Left to Right |
Bitwise exclusive OR |
^ |
Left to Right |
Bitwise inclusive OR |
| |
Left to Right |
Logical AND |
&& |
Left to Right |
Logical OR |
|| |
Left to Right |
Conditional |
?: |
Right to Left |
Assignment |
=, *=, /=, %=, +=, -=, &=, ^=, |=, <<=, >>= |
Right to Left |
Comma |
, |
Right to Left |
Unary & +, -, and * have higher precedence than the binary forms.
The Decision Control Structure - C Programming
C has three major decision making instructions—the if
statement, the if-else
statement, and the switch
statement.
1. The if Statement
C uses the keyword if to implement the decision control instruction. The general form of if statement looks like this:
//for single statement
if(condition)
statement;
//for multiple statement
if(condition)
{
block of statement;
}
The more general form is as follows:
//for single statement
if(expression)
statement;
//for multiple statement
if(expression)
{
block of statement;
}
Here the expression can be any valid expression including a relational expression. We can even use arithmetic expressions in the ifstatement. For example all the following if
statements are valid
if (3 + 2 % 5)
printf("This works");
The expression (3 + 2 % 5)
evaluates to 5 and since 5 is non-zero it is considered to be true. Hence the printf("This works");
gets executed.
2. The if-else Statement
The if statement by itself will execute a single statement, or a group of statements, when the expression following if evaluates to true. It does nothing when the expression evaluates to false. Can we execute one group of statements if the expression evaluates to true and another group of statements ifthe expression evaluates to false? Of course! This is what is the purpose of the else statement that is demonstrated as
if (expression)
{
block of statement;
}
else
statement;
Note
- The group of statements after the
if
upto and not including the else
is called an if block
. Similarly,the statements after the else
form the else block
.
- Notice that the
else
is written exactly below the if
. The statements in the if block
and those in the else block
have been indented to the right.
- Had there been only one statement to be executed in the
if block
and only one statement in the else block
we could have dropped the pair of braces.
- As with the
if
statement, the default scope of else
is also the statement immediately after the else
. To override this default scope a pair of braces as shown in the above "Multiple Statements within if" must be used.
3. Nested if-elses
If we write an entire if-else
construct within either the body of the if
statement or the body of an else
statement. This is called "nesting" of ifs
. This is demonstrated as -
if (expression1)
statement;
else
{
if (expression2)
statement;
else
{
block of statement;
}
}
4. The if-else Ladder/else-if Clause
The else-if
is the most general way of writing a multi-way decision.
if(expression1)
statement;
else if(expression2)
statement;
else if(expression3)
statement;
else if(expression4)
{
block of statement;
}
else
statement;
The expressions are evaluated in order; if an expressionis true, the "statement" or "block of statement" associated with it is executed, and this terminates the whole chain. As always, the code for each statement is either a single statement, or a group of them in braces. The last else
part handles the "none of the above" or default case where none of the other conditions is satisfied.
5. Switch Statements or Control Statements
The switch
statement is a multi-way decision that tests whether an expression matches one of a number of constant
integer values, and branches accordingly. The switch
statement that allows us to make a decision from the number of choices is called a switch
, or more correctlya switch-case-default
, since these three keywords go together to make up the switch
statement.
switch (expression)
{
case constant-expression:
statement1;
statement2;
break;
case constant-expression:
statement;
break;
...
default:
statement;
}
- In
switch…case
command, each case
acts like a simple label. A label determines a point in program which execution must continue from there. Switch statement will choose one of case
sections or labels from where the execution of the program will continue. The program will continue execution until it reaches break
command.
break
statements have vital rule in switch
structure. If you remove these statements, program execution will continue to next case sections and all remaining case sections until the end of switch
block will be executed (while most of the time we just want one case
section to be run).
default
section will be executed if none of the case sections match switch comparison.
6. switch Versus if-else Ladder
There are some things that you simply cannot do with a switch
.
These are:
- A float expression cannot be tested using a switch
- Cases can never have variable expressions (for example it is
wrong to say case a +3 :)
- Multiple cases cannot use same expressions.
The Loop Control Structure - C Programming
Sometimes we want some part of our code to be executed more than once. We can either repeat the code in our program or use loops instead. It is obvious that if for example we need to execute some part of code for a hundred times it is not practical to repeat the code. Alternatively we can use ourrepeating code inside a loop.
There are three methods for
, while
and do-while
which we can repeat a part of a program.
1. while loop
while loop is constructed of a condition or expression and a single command or a block of commands that must run
in a loop.
//for single statement
while(expression)
statement;
//for multiple statement
while(expression)
{
block of statement
}
The statements within the while
loopwould keep on getting executed till the condition being tested remains true. When the condition becomes false, the control passes to the first statement that follows the body of the while loop.
The general form of while
is as shown below:
initialise loop counter;
while (test loopcounter using a condition)
{
do this;
and this;
increment loopcounter;
}
Example
#include <stdio.h>
int main () {
int a = 10;
while( a < 20 ) {
printf("value of a: %d\n", a);
a++;
}
return 0;
}
When the above code is compiled and executed, it produces the following result −
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
value of a: 16
value of a: 17
value of a: 18
value of a: 19
2. for loop
for
loop is something similar to while
loop but it is more complex. for
loop is constructed from acontrol statement that determines how many times the loop will run and a command section. Command section is either a single command or a block of commands.
//for single statement
for(control statement)
statement;
//for multiple statement
for(control statement)
{
block of statement
}
Control statement itself has three parts:
for ( initialization; test condition; run every time command )
Initialization
part is performed only once at for
loop start. We can initialize a loop variable here.
Test condition
is the most important part of the loop. Loop will continue to run if this condition is valid (true). If the condition becomes invalid (false) then the loop will terminate.
Run every time command
section will be performed in every loop cycle. We use this part to reach the final condition for terminating the loop. For example we can increase or decrease loop variable’s value in a way that after specified number of cycles the loop condition becomes invalid and for
loop can terminate.
Example
#include <stdio.h>
int main () {
int a;
for( a = 10; a < 20; a = a + 1 ){
printf("value of a: %d\n", a);
}
return 0;
}
When the above code is compiled and executed, it produces the following result −
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
value of a: 16
value of a: 17
value of a: 18
value of a: 19
3. do-while loop
The while
and for
loops test the termination condition at the top. By contrast, the third loop in C, the do-while
, tests at the bottom after making each pass through the loop body; the body is always executed at least once.
The syntax of the do is
do
{
statements;
}while (expression);
The statement is executed, then expression
is evaluated. If it is true, statement is evaluated again, and so on. When the expression becomes false, the loop terminates. Experience shows that do-while
is much less used than while
and for
. A do-whileloop is used to ensure that the statements within the loop are executed at least once.
Example
#include <stdio.h>
int main () {
int a = 10;
do {
printf("value of a: %d\n", a);
a = a + 1;
}while( a < 20 );
return 0;
}
When the above code is compiled and executed, it produces the following result −
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
value of a: 16
value of a: 17
value of a: 18
value of a: 19
4. Break and Continue statement
We used break
statement in switch...case
structures in previouslly. We can also use
"break" statement inside loops to terminate a loop and exit it (with a specific condition).
In above example loop execution continues until either num>=20
or entered score is negative.
while (num<20)
{
printf("Enter score : ");
scanf("%d",&scores[num]);
if(scores[num]<0)
break;
}
Continue
statement can be used in loops. Like breakcommand continue
changes flow of a program. It does not terminate the loop however. It just skips the rest of current iteration of the loop and returns to starting point of the loop.
Example 1 (break)
#include <stdio.h>
int main () {
int a = 10;
while( a < 20 ) {
printf("value of a: %d\n", a);
a++;
if( a > 15) {
break;
}
}
return 0;
}
When the above code is compiled and executed, it produces the following result −
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
Example 2 (continue)
#include<stdio.h>
main()
{
while((ch=getchar())!='\n')
{
if(ch=='.')
continue;
putchar(ch);
}
}
In above example, program accepts all input but omits the '.' character from it. The text will be echoed as you enter it but the main output will be printed after you press the enter key (which is equal to inserting a "\n" character) is pressed. As we told earlier this is because getchar()
function is a buffered input function.
5. Goto and labels
C provides the infinitely-abusable goto
statement, and labels
to branch to. Formally, the goto statement is never necessary, and in practice it is almost always easy to write code without it. We have not used goto in this book.
Nevertheless, there are a few situations where gotos may find a place. The most common is to abandon processing in some deeply nested structure, such as breaking out of two or more loops at once. The break statement cannot be used directly since it only exits from the innermost loop. Thus:
for ( ... )
{
for ( ... )
{
...
if (disaster)
{
goto error;
}
}
}
...
error:
/* clean up the mess */
This organization is handy if the error-handling code is non-trivial, and if errors can occur in several places.
A label
has the same form as a variable name, and is followed by a colon. It can be attached to any statement in the same function as the goto. The scope of a label is the entire function.
Example
#include <stdio.h>
int main () {
int a = 10;
LOOP:do {
if( a == 15) {
a = a + 1;
goto LOOP;
}
printf("value of a: %d\n", a);
a++;
}while( a < 20 );
return 0;
}
When the above code is compiled and executed, it produces the following result −
value of a: 10
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 16
value of a: 17
value of a: 18
value of a: 19
Note - By uses of goto
, programs become unreliable, unreadable, and hard to debug. And yet many programmers find goto
seductive.
Array - C Programming
Arrays are structures that hold multiple variables of the same data type. The first element in the array is numbered 0, so the last element is 1 less than the size of the array. An array is also known as a subscripted variable. Before using an array its type and dimension must be declared.
1. Array Declaration
Like other variables an array needs to be declared so that the compiler will know what kind of an array and how large an array we want.
int marks[30] ;
Here, int
specifies the type of the variable, just as it does with ordinary variables and the word marks
specifies the name of the variable. The [30]
however is new. The number 30 tells how many elements of the type int
will be in our array. This number is often called the "dimension" of the array. The bracket ( [ ] )
tells the compiler that we are dealing with an array.
Let us now see how to initialize an array while declaring it. Following are a few examples that demonstrate this.
int num[6] = { 2, 4, 12, 5, 45, 5 } ;
int n[] = { 2, 4, 12, 5, 45, 5 } ;
float press[] = { 12.3, 34.2 -23.4, -11.3 } ;
2. Accessing Elements of an Array
Once an array is declared, let us see how individual elements in the array can be referred. This is done with subscript, the number in the brackets following the array name. This number specifies the element’s position in the array. All the array elements are numbered, starting with 0. Thus, marks [2] is not the second element of the array, but the third.
int valueOfThirdElement = marks[2];
3. Entering Data into an Array
Here is the section of code that places data into an array:
for(i = 0;i <= 29;i++)
{
printf("\nEnter marks ");
scanf("%d", &marks[i]);
}
The for
loop causes the process of asking for and receiving a student’s marks from the user to be repeated 30 times. The first time through the loop, i
has a value 0, so the scanf()
function will cause the value typed to be stored in the array element marks[0]
, the first element of the array. This process will be repeated until i
becomes 29. This is last time through the loop, which is a good thing, because there is no array element like marks[30]
.
In scanf()
function, we have used the "address of" operator (&) on the element marks[i]
of the array. In so doing, we are passing the address of this particular array element to the scanf()
function, rather than its value; which is what scanf()
requires.
4. Reading Data from an Array
The balance of the program reads the data back out of the array and uses it to calculate the average. The for loop is much the same, but now the body of the loop causes each student’s marks to be added to a running total stored in a variable called sum. When all the marks have been added up, the result is divided by 30, the number of students, to get the average.
for ( i = 0 ; i <= 29 ; i++ )
sum = sum + marks[i] ;
avg = sum / 30 ;
printf ( "\nAverage marks = %d", avg ) ;
5. Advantages of using an Array
- Similar data types can be grouped together under one name.
for ex.- int a=1;int b=2;int c=3;int d=4;int e=5;
could be represented as- int X[5]={1,2,3,4,5};
- It allows random accessing of elements using indices which saves a huge time.
- It can be used to implement other data structures like- stack,queues etc.
- We can represent 2-D matrices using array.
6. Disadvantages of using an Array
- It is mandatory to determine the size of array to store the elements in it.
- As array elements are stored at consecutive memory locations,so insertion and deletion of an element is difficult/time consuming.
- There is a certain chance of memory wastage/shortage.
- Array size is static in nature so size of array cannot be altered.
7. Multidimensional arrays
The simplest form of multidimensional array is the two-dimensional array. A two-dimensional array is, in essence, a list of one-dimensional arrays. To declare a two-dimensional integer array of size [x][y], you would write something as follows −
type arrayName [x][y];
Multidimensional arrays may be initialized by specifying bracketed values for each row. Following is an array with 3 rows and each row has 4 columns.
int a[3][4] = {
{0, 1, 2, 3} ,
{4, 5, 6, 7} ,
{8, 9, 10, 11}
};
The nested braces, which indicate the intended row, are optional. The following initialization is equivalent to the previous example −
int a[3][4] = {0,1,2,3,4,5,6,7,8,9,10,11};
8. Example
8.1 Simple Array
Let us try to write a program to find average marks obtained by a
class of 30 students in a test.
#include<stdio.h>
main()
{
int avg, i, sum=0;
int marks[30] ;
for ( i = 0 ; i <= 29 ; i++ )
{
printf ( "\nEnter marks " ) ;
scanf ( "%d", &marks[i] ) ;
}
for ( i = 0 ; i <= 29 ; i++ )
sum = sum + marks[i] ;
avg = sum / 30 ;
printf ( "\nAverage marks = %d", avg ) ;
}
8.2 Two-Dimensional Array
#include <stdio.h>
main () {
int a[5][2] = { {0,0}, {1,2}, {2,4}, {3,6},{4,8}};
int i, j;
for ( i = 0; i < 5; i++ ) {
for ( j = 0; j < 2; j++ ) {
printf("a[%d][%d] = %d\n", i,j, a[i][j] );
}
}
return 0;
}
Strings - C Programming
1. What is String?
Strings are arrays of characters. Each member of array contains one of characters in the string.
Example
#include<stdio.h>
main()
{
char name[20];
printf("Enter your name : ");
scanf("%s",name);
printf("Hello, %s , how are you ?\n",name);
}
Output Results:
Enter your name : Vineet
Hello, Vineet, how are you ?
If user enters "Vineet" then the first member of array will contain 'V' , second cell will contain 'i' and so on. C determines end of a string by a zero value character. We call this character as NULL
character and show it with \0
character. (It's only one character and its value is 0, however we show it with two characters to remember it is a character type, not an integer).
Equally we can make that string by assigning character values to each member.
name[0]='B';
name[1]='r';
name[2]='i';
name[3]='a';
name[4]='n';
name[5]='\0';
As we saw in above example placeholder for string variables is %s
. Also we will not use a &
sign for receiving string values.
2. Point to Note
While entering the string using scanf()
we must be cautious about
two things:
- The length of the string should not exceed the dimension of the character array. This is because the C compiler doesn’t perform bounds checking on character arrays.
scanf()
is not capable of receiving multi-word strings. Therefore names such as "Vineet Choudhary" would be unacceptable. The way to get around this limitation is by using the function gets()
.The usage of functions gets()
and its counter part puts()
is shown below.
#include<stdio.h>
main( )
{
char name[25] ;
printf ("Enter your full name ") ;
gets (name) ;
puts ("Hello!") ;
puts (name) ;
}
And here is the output...
Enter your name Vineet Choudhary
Hello!
Vineet Choudhary
The program and the output are self-explanatory except for the fact that, puts()
can display only one string at a time (hence the use of two puts()
in the program above). Also, on displaying a string, unlike printf()
, puts()
places the cursor on the next line. Though gets()
is capable of receiving only one string at a time, the plus point with gets()
is that it can receive a multi-word string.
If we are prepared to take the trouble we can make scanf()
accept multi-word strings by writing it in this manner:
char name[25] ;
printf ("Enter your full name ") ;
scanf ("%[^\n]s", name) ;
Though workable this is the best of the ways to call a function, you would agree.
3. Standard Library String Functions
With every C compiler a large set of useful string handling library functions are provided in string.h
file.
strlen
- Finds length of a string
strlwr
- Converts a string to lowercase
strupr
- Converts a string to uppercase
strcat
- Appends one string at the end of another
strncat
- Appends first n characters of a string at the end of
another
strcpy
- Copies a string into another
strncpy
- Copies first n characters of one string into another
strcmp
- Compares two strings
strncmp
- Compares first n characters of two strings
strcmpi
- Compares two strings without regard to case ("i" denotes
that this function ignores case)
stricmp
- Compares two strings without regard to case (identical to
strcmpi)
strnicmp
- Compares first n characters of two strings without regard
to case
strdup
- Duplicates a string
strchr
- Finds first occurrence ofa given character in a string
strrchr
- Finds last occurrence ofa given character in a string
strstr
- Finds first occurrence of a given string in another string
strset
- Sets all characters ofstring to a given character
strnset
- Sets first n characters ofa string to a given character
strrev
- Reverses string
4. Example
The following example uses some of the above-mentioned functions −
#include <stdio.h>
#include <string.h>
int main () {
char str1[12] = "Hello";
char str2[12] = "World";
char str3[12];
int len ;
strcpy(str3, str1);
printf("strcpy( str3, str1) : %s\n", str3 );
strcat( str1, str2);
printf("strcat( str1, str2): %s\n", str1 );
len = strlen(str1);
printf("strlen(str1) : %d\n", len );
return 0;
}
When the above code is compiled and executed, it produces the following result −
strcpy( str3, str1) : Hello
strcat( str1, str2): HelloWorld
strlen(str1) : 10
5. Examples
EXAMPLE STATEMENT FOR STRING IN C LANGUAGE |
1. Check whether two strings are anagrams or not
#include <stdio.h>
int check_anagram(char [], char []);
void main()
{
char a[100], b[100];
int flag;
clrscr();
printf("Enter first string\n");
gets(a);
printf("Enter second string\n");
gets(b);
flag = check_anagram(a, b);
if (flag == 1)
printf("\"%s\" and \"%s\" are anagrams.\n", a, b);
else
printf("\"%s\" and \"%s\" are not anagrams.\n", a, b);
getch();
}
int check_anagram(char a[], char b[])
{
int first[26] = {0}, second[26] = {0}, c = 0;
while (a[c] != '\0')
{
first[a[c]-'a']++;
c++;
}
c = 0;
while (b[c] != '\0')
{
second[b[c]-'a']++;
c++;
}
for (c = 0; c < 26; c++)
{
if (first[c] != second[c])
return 0;
}
return 1;
}
|
2. Find a frequenct of character in string
#include <stdio.h>
#include <string.h>
#include <conio.h>
void find_frequency(char [], int []);
void main()
{
char string[100];
int c, count[26] = {0};
clrscr();
printf("Input a string\n");
gets(string);
find_frequency(string, count);
printf("Character Count\n");
for (c = 0 ; c < 26 ; c++)
{
printf("%c \t %d\n", c + 'a', count[c]);
}
getch();
}
void find_frequency(char s[], int count[]) {
int c = 0;
while (s[c] != '\0') {
if (s[c] >= 'a' && s[c] <= 'z' )
count[s[c]-'a']++;
c++;
}
}
|
3. Insert substring into a string
#include <stdio.h>
#include <conio.h>
#include <string.h>
#include <stdlib.h>
void insert_substring(char*, char*, int);
char* substring(char*, int, int);
void main()
{
char text[100], substring[100];
int position;
clrscr();
printf("Enter some text\n");
gets(text);
printf("Enter the string to insert\n");
gets(substring);
printf("Enter the position to insert\n");
scanf("%d", &position);
insert_substring(text, substring, position);
printf("%s\n",text);
getch();
}
void insert_substring(char *a, char *b, int position)
{
char *f, *e;
int length;
length = strlen(a);
f = substring(a, 1, position - 1 );
e = substring(a, position, length-position+1);
strcpy(a, "");
strcat(a, f);
free(f);
strcat(a, b);
strcat(a, e);
free(e);
}
char *substring(char *string, int position, int length)
{
char *pointer;
int c;
pointer = malloc(length+1);
if( pointer == NULL )
exit(EXIT_FAILURE);
for( c = 0 ; c < length ; c++ )
*(pointer+c) = *((string+position-1)+c);
*(pointer+c) = '\0';
return pointer;
}
|
4. Substring from a position to length
#include <stdio.h>
#include <conio.h>
void main()
{
char string[1000], sub[1000];
int position, length, c = 0;
clrscr();
printf("Input a string\n");
gets(string);
printf("Enter the position and length of substring\n");
scanf("%d%d", &position, &length);
while (c < length) {
sub[c] = string[position+c-1];
c++;
}
sub[c] = '\0';
printf("Required substring is \"%s\"\n", sub);
getch();
}
|
5. Check if a string is a subsequence of another string
#include <stdio.h>
#include <string.h>
#include <conio.h>
int check_subsequence (char [], char[]);
void main () {
int flag;
char s1[1000], s2[1000];
clrscr();
printf("Input first string\n");
gets(s1);
printf("Input second string\n");
gets(s2);
if (strlen(s1) < strlen(s2))
flag = check_subsequence(s1, s2);
else
flag = check_subsequence(s2, s1);
(flag)?printf("YES\n"):printf("NO\n");
getch();
}
int check_subsequence (char a[], char b[]) {
int c, d;
c = d = 0;
while (a[c] != '\0') {
while ((a[c] != b[d]) && b[d] != '\0') {
d++;
}
if (b[d] == '\0')
break;
d++;
c++;
}
if (a[c] == '\0')
return 1;
else
return 0;
}
|
6. Delete vowels from a string
|
#include <stdio.h>
#include <string.h>
#include <conio.h>
int check_vowel(char);
void main()
{
char s[100], t[100];
int i, j = 0;
clrscr();
printf("Enter a string to delete vowels\n");
gets(s);
for(i = 0; s[i] != '\0'; i++) {
if(check_vowel(s[i]) == 0) {
t[j] = s[i];
j++;
}
}
t[j] = '\0';
strcpy(s, t);
printf("String after deleting vowels: %s\n", s);
getch();
}
int check_vowel(char c)
{
switch(c) {
case 'a':
case 'A':
case 'e':
case 'E':
case 'i':
case 'I':
case 'o':
case 'O':
case 'u':
case 'U':
return 1;
default:
return 0;
}
}
Functions - C Programming
1. What is a Function?
A function is combined of a block of code that can be called or used anywhere in the program by calling the name. Body of a function starts with {
and ends with }
. This is similar to the main function. Example below shows how we can write a simple function.
#include<stdio.h>
myfunc();
main()
{
myfunc();
}
myfunc()
{
printf("Hello, this is a test\n");
}
In above example we have put a section of program in a separate function. Function body can be very complex though. After creating a function we can call it using its name. Functions can also call each other. A function can even call itself.
By the way pay attention to function prototypes
section. In some C compilers we are needed to introduce the functions we are creating in a program above the program. Introducing a function is being called function prototype
.
Note:
- Any C program contains at least one function.
- If a program contains only one function, it must be
main()
.
- If a C program contains more than one function, then one (and only one) of these functions must be
main()
, because program execution always begins with main()
.
- There is no limit on the number of functions that might be present in a C program.
- Each function in a program is called in the sequence specified by the function calls in
main()
.
- After each function has done its thing, control returns to
main()
. When main()
runs out of function calls, the program ends.
2. Why Use Functions
Why write separate functions at all? Why not squeeze the entire
logic into one function, main()
? Two reasons:
- Writing functions avoids rewriting the same code over and over.
- Using functions it becomes easier to write programs and keep track of what they are doing. If the operation of a program can be divided into separate activities, and each activity placed in a different function, then each could be written and checked more or less independently. Separating the code into modular functions also makes the program easier to design and understand.
What is the moral of the story? Don’t try to cram the entire logic in one function. It is a very bad style of programming. Instead, break a program into small units and write functions for each of these isolated subdivisions. Don’t hesitate to write functions that are called only once. What is important is that these functions perform some logically isolated task.
3. Function arguments
Functions are able to accept input parameters in the form of variables. These input parameter variables
can then be used in function body.
#include<stdio.h>
sayhello(int count);
main()
{
sayhello(4);
}
sayhello(int count)
{
int c;
for(c=0;c<count;c++)
printf("Hello\n");
}
In above example we have called sayhello()
function with the parameter 4
. This function receives an input value and assigns it to count
variable before starting execution of function body. sayhello()
function will then print a hello message count
times on the screen.
4. Function return values
In mathematics we generally expect a function to return a value. It may or may not accept arguments but it always returns a value.
This return
value has a type as other values in C. It can be int
, float
, char
or anything else. Type of this return
value determines type of your function.
Default type of function is int
or integer. If you do not indicate type of function that you use, it will be of type int
. As we told earlier every function must return a value. We do this with return
command.
int sum()
{
int a,b,c;
a=1;
b=4;
c=a+b;
reurn c;
}
Above function returns the value of variable c as the return value of function. We can also use expressions in return command. For example we can replace two last lines of function with return a+b;
If you forget to return a value in a function you will get a warning message in most of C compilers. This message will warn you that your function must return a value. Warnings do not stop program execution but errors stop it.
In our previous examples we did not return any value in our functions. For example you must return a value in main() function.
main()
{
.
.
.
return 0;
}
Default return value for an int
type function is 0. If you do not insert return 0
or any other value in your main()
function a 0 value will be returned automatically. If you are planning to return an int
value in your function, it is seriously preferred to mention the return value in your function header and make.
5. void return value
There is another void
type of function in C language. Void type function is a function that does not return
a value. You can define a function that does not need a return value as void
.
void test ()
{
}
void
functions cannot be assigned to a variable because it does not return value. So you cannot write:
a=test();
Using above command will generate an error.
6. Recursive Function
In C, it is possible for the functions to call themselves. A function is called recursive
if a statement within the body of a function calls the same function.
Following is the recursive function to calculate the factorial value.
#include<stdio.h>
int rec(int);
main()
{
int a, fact ;
printf("\nEnter any number ");
scanf ("%d", &a);
fact = rec(a);
printf ("Factorial value = %d", fact);
}
int rec (int x)
{
int f;
if (x == 1)
return (1);
else
f = x * rec (x - 1) ;
return (f) ;
}
Output-
Enter any number 5
Factorial value = 120
Let us understand this recursive factorial function.
what happens is,
rec(5) returns(5 * rec(4),
which returns (4 * rec(3),
which returns (3 * rec(2),
which returns (2 * rec(1),
which returns (1)))))
Foxed? Well, that is recursion for you in its simplest garbs. I hope you agree that it’s difficult to visualize how the control flows from one function call to another. Possibly Following figure would make things a bit clearer.
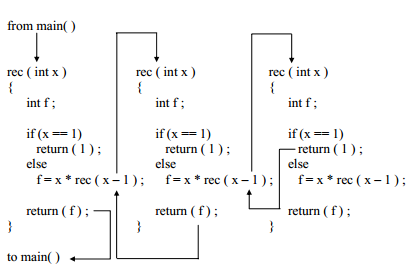
7. Multiple Parameters
In fact you can use more than one argument in a function. Following example will show you how you can do this.
#include<stdio.h>
int min(int a,int b);
main()
{
int m;
m=min(3,6);
printf("Minimum is %d",m);
return 0;
}
int min(int a,int b)
{
if(a<b)
return a;
else
return b;
}
As you see you can add your variables to arguments list easily.
8. Call by value
C programming language function calls, use call by value method. Let’s see an example to understand this subject better.
#include<stdio.h>
void test(int a);
main()
{
int m;
m=2;
printf("\nM is %d",m);
test(m);
printf("\nM is %d\n",m);
return 0;
}
void test(int a)
{
a=5;
}
In main()
function, we have declared a variable m
. We have assigned value 2
to m
. We want to see if function call is able to change value of m
as we have modified value of incoming parameter inside test()
function.
Program output result is:
M is 2
M is 2
So you see function call has not changed the value of argument. This s
is because function-calling method only sends value of variable m
to the function and it does not send variable itself. Actuallyit places value of variable m
in a memory location called stack and then function retrieves the value without having access to main variable itself. This is why we call this method of calling "call by value".
9. Call by reference
There is another method of sending variables being called "Call by reference". This second method enables function to modify value of argument variables used in function call.
We will first see an example and then we will describe it.
#include<stdio.h>
void test(int *ptr);
main()
{
int m;
m=2;
printf("\nM is %d",m);
test(&m);
printf("\nM is %d\n",m);
return 0;
}
void test(int *ptr)
{
printf("\nModifying the value inside memory address%ld",&m);
*ptr=5;
}
To be able to modify the value of a variable used as an argument in a function, function needs to know memory address of the variable (where exactly the variable is situated in memory).
In C programming language &
operator gives the address at which the variable is stored. For example if m
is a variable of type int
then &m
will give us the starting memory address of our variable.We call this resulting address a pointer
.
ptr=&m;
In above command ptr
variable will contain memory address of variable m
. This method is used in some of standard functions in C. For example scanf
function uses this method to be able to receive values from console keyboard and put it in a variable. In fact it places received value in memory location of the variable used in function. Now we understand the reason why we add &
sign before variable names in scanf
variables.
scanf(“%d”,&a);
Now that we have memory address of variable we must use an operator that enables us to assign a value or access to value stored in that address.
As we told, we can find address of variable a
using &
operator.
ptr=&a;
Now we can find value stored in variable a
using *
operator:
val=*ptr;
We can even modify the value inside the address:
*ptr=5;
Let’s look at our example again. We have passed pointer (memory address) to function. Now function is able to modify value stored inside variable. If yourun the program, you will get these results:
M is 2
Modifying the value inside memory address 2293620
M is 5
So you see this time we have changed value of an argument variable inside the called function (by modifying the value inside the memory location of our main variable).
Pointers - C Programming
1. What is a Pointer?
A pointer is a variable that contains the address of a variable. The main thing is that once you can talk about the address of a variable, you'll then be able to goto that address and retrieve the data stored in it.
2. C Pointer Syntax
Pointers require a bit of new syntax because when you have a pointer, you need the ability to both request the memory location it stores and the value stored at that memory location. Moreover, since pointers are some what special, you need to tell the compiler when you declare your pointer variable that the variable is a pointer, and tell the compiler what type of memory it points to.
The pointer declaration looks like this:
<variable_type> *<name>;
For example, you could declarea pointer that stores the address of an integer with the following syntax:
int *points_to_integer;
Notice the use of the *. This is the key to declaring a pointer; if you add it directly before the variable name, it will declare the variable to be a pointer.
3. Pointer Notation
Consider the declaration,
int i = 3;
This declaration tells the C compiler to:
- Reserve space in memory to hold the integer value.
- Associate the name
i
with this memory location.
- Store the value 3 at this location.
We may represent i’s
location in memory by the following memory map.
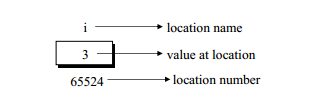
We see that the computer has selected memory location 65524 as the place to store the value 3. The location number 65524 is not a number to be relied upon, because some other time the computer may choose a different location for storing the value 3. The important point is, i’s address in memory is a number. We can print this address number through the following program:
#include<stdio.h>
main()
{
int i = 3 ;
printf("\nAddress of i = %u",&i);
printf("\nValue of i = %d",i);
}
The output of the above program would be:
Address of i = 65524
Value of i = 3
Look at the first printf()
statement carefully. &
used in this statement is C’s "address of" operator. The expression &i
returns the address of the variable i
, which in this case happens to be 65524. Since 65524 represents an address, there is no question of a sign being associated with it. Hence it is printed out using %u
, which is a format specifier for printing an unsigned integer. We have been using the &
operator all the time in the scanf()
statement.
The other pointer operator available in C is *
, called "value at address" operator. It gives the value stored at a particular address. The "value at address" operator is also called "indirection" operator.
Observe carefully the output of the following program:
#include<stdio.h>
main()
{
int i = 3 ;
printf("\nAddress of i = %u",&i);
printf("\nValueof i = %d",i);
printf("\nValue of i = %d",*(&i));
}
The output ofthe above programwould be:
Address of i = 65524
Value of i = 3
Value of i = 3
Note that printing the value of *(&i)
is same as printing the value of i
. The expression &i
gives the address of the variable i. This address can be collected in a variable, by saying,
j = &i ;
But remember that j
is not an ordinary variable like any other integer variable. It is a variable that contains the address of other variable (i
in this case). Since j
is a variable the compiler must provide it space in the memory. Once again, the following memory map would illustrate the contents of i
and j.
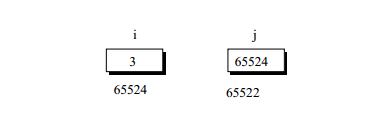
As you can see, i's
value is 3 and j's
value is i's
address. But wait, we can’t use j
in a program without declaring it. And since j
is a variable that contains the address of i
, it is declared as,
int *j ;
This declaration tells the compiler that j
will be used to store the address of an integer value. In other words j
points to an integer. How do we justify the usage of *
in the declaration,
int *j ;
Let us go by the meaning of *
. It stands for "value at address". Thus, int *j
would mean, the value at the address contained in j
is an int.
4. Example
Here is a program that demonstrates the relationships we have been discussing.
#include<stdio.h>
main( )
{
int i = 3 ;
int *j ;
j = &i ;
printf ("\nAddress of i = %u",&i) ;
printf ("\nAddress of i = %u",j) ;
printf ("\nAddress of j = %u",&j) ;
printf ("\nValue of j = %u",j) ;
printf ("\nValue of i = %d",i) ;
printf ("\nValue of i = %d",*(&i)) ;
printf ("\nValue of i = %d",*j) ;
}
The output of the above program would be:
Address of i = 65524
Address of i = 65524
Address of j = 65522
Value of j = 65524
Value of i = 3
Value of i = 3
Value of i = 3
5. Examples
EXAMPLE STATEMENT FOR POINTER IN C LANGUAGE
|
1. Add numbers using call by reference
#include <stdio.h>
#include <conio.h>
long add(long *, long *);
void main()
{
long first, second, sum;
clrscr();
printf("Input two integers to add\n");
scanf("%ld%ld", &first, &second);
sum = add(&first, &second);
printf("(%ld) + (%ld) = (%ld)\n", first, second, sum);
getch();
}
long add(long *x, long *y) {
long sum;
sum = *x + *y;
return sum;
}
|
2. Multilevel Pointer [With Explanation]
#include<stdio.h>
#include<conio.h>
void main(){
int s=2,*r=&s,**q=&r,***p=&q;
clrscr();
printf("%d",p[0][0][0]);
getch();
}
|
3. Sum of array elements using pointers
|
#include<stdio.h>
#include<conio.h>
void main() {
int numArray[10];
int i, sum = 0;
int *ptr;
printf("\nEnter 10 elements : ");
for (i = 0; i < 10; i++)
{
scanf("%d", &numArray[i]);
}
ptr = numArray;
for (i = 0; i < 10; i++)
{
sum = sum + *ptr;
ptr++;
}
printf("The sum of array elements : %d", sum);
getch();
}
Structures - C Programming
A structure is a collection of one or more variables, possibly of different types, grouped together under a single name for convenient handling.
1. Declaring a Structure
The general form of a structure declaration statement is given below:
struct <structure name>
{
structure element 1;
structure element 2;
structure element 3;
......
......
structure element n;
};
Once the new structure data type has been defined one or more variables can be declared to be of that type.
For example the variables b1, b2, b3 can be declared to be of the type struct book,
struct book
{
char name;
float price;
int pages;
};
as,
struct book b1, b2, b3;
This statement sets aside space in memory. It makes available space to hold all the elements in the structure—in this case, 7 bytes — one for name, four for price and two for pages. These bytes are always in adjacent memory locations.
Like primary variables and arrays, structure variables can also be initialized where they are declared. The format used is quite similar to that used to initiate arrays.
struct book
{
char name[10];
float price;
int pages;
};
struct book b1 = { "Basic", 130.00, 550 } ;
struct book b2 = { "Physics", 150.80, 800 } ;
2. Accessing Structure Elements
In arrays we can access individual elements of an array using a subscript. Structures use a different scheme. They use a dot (.
) operator. So to refer to pages
of the structure defined in book structure we have to use,
b1.pages
Similarly, to refer to price
we would use,
b1.price
Note that before the dot there must always be a structure variable and after the dot there must always be a structure element.
3. Example
The following example illustrates the use of this data type.
#include<stdio.h>
main()
{
struct book
{
char name;
float price;
int pages;
};
struct book b1, b2, b3 ;
printf("\nEnter names, prices & no. of pages of 3 books\n");
scanf("%c %f %d", &b1.name, &b1.price, &b1.pages);
scanf("%c %f %d", &b2.name, &b2.price, &b2.pages);
scanf("%c %f %d", &b3.name, &b3.price, &b3.pages);
printf("\n\nAnd this is what you entered");
printf("\n%c %f %d", b1.name, b1.price, b1.pages);
printf("\n%c %f %d", b2.name, b2.price, b2.pages);
printf("\n%c %f %d", b3.name, b3.price, b3.pages);
}
And here is the output...
Enter names, prices and no. of pages of 3 books
A 100.00 354
C 256.50 682
F 233.70 512
And this is what you entered
A 100.000000 354
C 256.500000 682
F 233.700000 512
4. Structures as Function Arguments
You can pass a structure as a function argument in the same way as you pass any other variable.
#include <stdio.h>
#include <string.h>
struct Books {
char title[50];
char author[50];
char subject[100];
int book_id;
};
void printBook( struct Books book );
int main( ) {
struct Books Book1;
struct Books Book2;
strcpy( Book1.title, "C Programming");
strcpy( Book1.author, "Developer Insider");
strcpy( Book1.subject, "C Programming Tutorial");
Book1.book_id = 6495407;
strcpy( Book2.title, "C++ Programming");
strcpy( Book2.author, "Developer Insider");
strcpy( Book2.subject, "C++ Programming Tutorial");
Book2.book_id = 6495700;
printBook( Book1 );
printBook( Book2 );
return 0;
}
void printBook( struct Books book ) {
printf( "Book title : %s\n", book.title);
printf( "Book author : %s\n", book.author);
printf( "Book subject : %s\n", book.subject);
printf( "Book book_id : %d\n", book.book_id);
}
When the above code is compiled and executed, it produces the following result −
Book title : C Programming
Book author : Developer Insider
Book subject : C Programming Tutorial
Book book_id : 6495407
Book title : C++ Programming
Book author : Developer Insider
Book subject : C++ Programming Tutorial
Book book_id : 6495700
5. Summary
- A structure is usually used when we wish to store dissimilar data together.
- Structure elements can be accessed through a structure variable using a dot (
.
) operator.
- Structure elements can be accessed through a pointer to a structure using the arrow (
->
) operator.
- All elements of one structure variable can be assigned to another structure variable using the assignment (
=
) operator.
- It is possible to pass a structure variable to a function either by value or by address.
- It is possible to create an array of structures
6. Examples
EXAMPLE STATEMENT FOR STRUCTURE AND UNION IN C LANGUAGE |
1. Calculate Size of Structure
|
#include<stdio.h>
struct stud {
int roll;
char name[10];
int marks;
};
int main() {
int size;
struct stud s;
size = sizeof(s);
printf("nSize of Structure : %d", size);
return(0);
}
2. Use structure within union
|
#include<stdio.h>
#include<conio.h>
void main() {
struct student {
char name[30];
char sex;
int rollno;
float percentage;
};
union details {
struct student st;
};
union details set;
clrscr();
printf("Enter details:");
printf("\nEnter name : ");
scanf("%s", set.st.name);
printf("\nEnter roll no : ");
scanf("%d", &set.st.rollno);
flushall();
printf("\nEnter sex : ");
scanf("%c", &set.st.sex);
printf("\nEnter percentage :");
scanf("%f", &set.st.percentage);
printf("\nThe student details are : \n");
printf("\name : %s", set.st.name);
printf("\nRollno : %d", set.st.rollno);
printf("\nSex : %c", set.st.sex);
printf("\nPercentage : %f", set.st.percentage);
getch();
}
3. Find the size of a union
|
#include <stdio.h>
#include <conio.h>
void main()
{
union sample
{
int m;
float n;
char ch;
};
union sample u;
clrscr();
printf("The size of union =%d\n", sizeof(u));
u.m = 25;
printf("%d %f %c\n", u.m, u.n,u.ch);
u.n = 0.2;
printf("%d %f %c\n", u.m, u.n,u.ch);
u.ch = 'p';
printf("%d %f %c\n", u.m, u.n,u.ch);
getch();
}
Files Handling - C Programming
1. File Pointers
There are many ways to use files in C. The most straight forward use of files is via a file pointer.
FILE *fp;
fp
is a pointer to a file.
The type FILE
, is not a basic type, instead it is defined in the header file stdio.h
, this file must be included in your program.
2. Opening a File
fp = fopen(filename, mode);
The filename and mode are both strings.
The mode can be
r
- read
w
- write, overwrite file if it ex ists
a
- write, but append instead of overwrite
r+
- read & write, do not destroy file if it exists
w+
- read & write, but overwrite file if it exists
a+
- read & write, but append instead of overwrite
b
- may be appended to any of the above to force the file to be opened in binary mode rather than text mode
fp = fopen("data.dat","a");
- will open the disk file data.dat for writing, and any information written will be appended to the file.
The following useful table from the ANSI C Rationale lists the different actions and requirements of the different modes for opening a file:
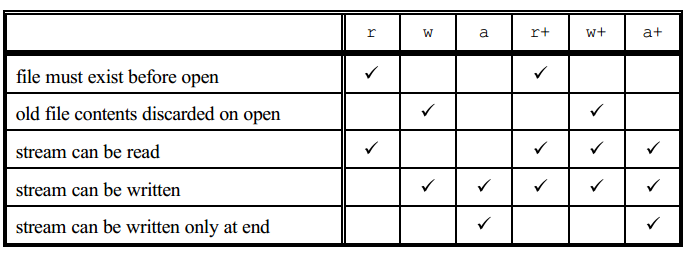
fopen
returns NULL
if the file could not be opened in the mode requested. The returned value should be checked before any attempt is made to access the file. The following code shows how the value returned by fopen might be checked. When the file cannot be opened a suitable error message is printed and the program halted. In most situations this would be inappropriate, instead the user should be given the chance of re-entering the file name.
#include <stdio.h>
int main()
{
char filename[80];
FILE *fp;
printf("File to be opened? ");
scanf("%79s", filename);
fp = fopen(filename,"r");
if (fp == NULL)
{
fpri ntf(stderr, "Unable to open file %s\n", filename);
return 1;
}
return 0;
}
Sequential file access is performed with the following library functions.
fprintf(fp, formatstring , ...)
- print to a file
fscanf(fp, formatstring , ...)
- read from a file
getc(fp)
- get a character from a file
putc(c, fp)
- put a character in a file
ungetc(c, fp)
- put a character back onto a file (only one character is guaranteed to be able to be pushed back)
fopen( filename , mode)
- open a file
fclose(fp)
- close a file
The standard header file stdio.h defines three file pointer constants, stdin
,stdout
and stderr
for the standard input, output and error streams. It is considered good practice to write error messages to the standard error stream.
Use the fprintf()
function to do this:
fprintf(stderr,"ERROR: unable to open file %s\n", filename);
The functions fscanf()
and getc()
are used for sequential access to the file, that is subsequent reads will read the data following the data just read, and eventually you will reach the end of the file. If you want to move forwards and backwards through the file, or jump to a specific offset from the beginning, end or current location use the fseek()
function (though the file mustbe opened in binary mode). The function ftell()
reports the current offset from the beginning of the file.
If you wish to mix reading and writing to the same file, each switch from reading to writing (or vice versa) mustbe preceded by a call to fseek()
, fsetpos()
, rewind()
or fflush()
. If it is required to stay at the same position in the file then use fflush()
or fseek(fp,0L,SEEK_CUR)
which will move 0 bytes from the current position!
3. Examples
EXAMPLE STATEMENT FOR FILES HANDLING IN C LANGUAGE
|
1. Illustrate as to how the data stored on the disk is read
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
void main()
{
FILE *fptr;
char filename[15];
char ch;
clrscr();
printf("Enter the filename to be opened\n");
gets(filename);
fptr = fopen (filename, "r");
if (fptr == NULL)
{
printf("Cannot open file\n");
exit(0);
}
ch = fgetc(fptr);
while (ch != EOF)
{
printf ("%c", ch);
ch = fgetc(fptr);
}
fclose(fptr);
getch();
}
|
2. Write static data in file and read from file |
#include<stdio.h>
#include<conio.h>
struct Student {
int roll;
char name[12];
int percent;
} s1 = { 96, "VINEET", 76 };
void main() {
FILE *fp;
struct Student s2;
clrscr();
fp = fopen("ip.txt", "w");
fwrite(&s1, sizeof(s1), 1, fp);
fclose(fp);
fp = fopen("ip.txt", "r");
fread(&s2, sizeof(s2), 1, fp);
fclose(fp);
printf("\nRoll : %d", s2.roll);
printf("\nName : %s", s2.name);
printf("\nPercent : %d", s2.percent);
getch();
}
Command Line Arguments - C Programming
he arguments that we pass on to main()
at the command prompt are called command line arguments. The full declaration of main looks like this:
int main (int argc, char *argv[])
The function main()
can have two arguments, traditionally named as argc
and argv
. Out of these, argv
is an array of pointers to strings and argc
is an int
whose value is equal to the number of strings to which argv
points. When the program is executed, the strings on the command line are passed to main()
. More precisely, the strings at the command line are stored in memory and address of the first string is stored in argv[0]
, address of the second string is stored in argv[1]
and so on. The argument argc
is set to the number of strings given on the command line.
For example
In our sample program, if at the command prompt we give,
filecopy PR1.C PR2.C
then,
argc
would contain 3
argv[0]
- would contain base address of the string "filecopy"
argv[1]
- would contain base address of the string "PR1.C"
argv[2]
- would contain base address of the string "PR2.C"
Following is a simple example which checks if there is any argument supplied from the command line and take action accordingly −
#include <stdio.h>
int main( int argc, char *argv[] ) {
if( argc == 2 )
{
printf("The argument supplied is %s\n", argv[1]);
}
else if( argc > 2 )
{
printf("Too many arguments supplied.\n");
}
else
{
printf("One argument expected.\n");
}
}
When the above code is compiled and executed with single argument, it produces the following result.
argumenttest.exe testing
The argument supplied is testing
If you are using Turbo C++ compiler, checkout this tutorial - How to Pass Command Line Arguments in Turbo C++
Results of Parsing Command Lines
The following table shows example input and expected output, demonstrating the rules in the preceding list.
COMMAND-LINE INPUT | ARGV[1] | ARGV[2] | ARGV[3] |
"abc" d e |
abc |
d |
e |
a\\b d"e f"g h |
a\\b |
de fg |
h |
a\\\"b c d |
a\"b |
c |
d |
a\\\\"b c" d e |
a\\b c |
d |
e
|
Type Casting - C Programming
Type casting refers to changing an variable of one data type into another. The compiler will automatically change one type of data into another if it makes sense. For instance, if you assign an integer value to a floating-point variable, the compiler will convert the int to a float. Casting allows you to make this type conversion explicit, or to force it when it wouldn’t normally happen.
Type conversion in c can be classified into the following two types:
1. Implicit Type Conversion
When the type conversion is performed automatically by the compiler without programmers intervention, such type of conversion is known as implicit type conversion or type promotion.
int x;
for(x=97; x<=122; x++)
{
printf("%c", x);
}
2. Explicit Type Conversion
The type conversion performed by the programmer by posing the data type of the expression of specific type is known as explicit type conversion. The explicit type conversion is also known as type casting.
Type casting in c is done in the following form:
(data_type)expression;
where, data_type is any valid c data type, and expression may be constant, variable or expression.
For example,
int x;
for(x=97; x<=122; x++)
{
printf("%c", (char)x);
}
The following rules have to be followed while converting the expression from one type to another to avoid the loss of information:
- All integer types to be converted to float.
- All float types to be converted to double.
- All character types to be converted to integer.
Example
Consider the following code:
int x=7, y=5 ;
float z;
z=x/y;
If we want to get the exact value of 7/5 then we need explicit casting from int to float:
int x=7, y=5;
float z;
z = (float)x/(float)y;
Storage class specifiers are the keywords which can appear next to the top-level type of a declaration. The use of these keywords affects the storage duration and linkage of the declared object, depending on whether it is declared at file scope or at block scope:
1. auto
This storage class denotes that an identifier has automatic storage duration. This means once the scope in which the identifier was defined ends, the object denoted by the identifier is no longer valid.
Since all objects, not living in global scope or being declared static, have automatic storage duration by default when defined, this keyword is mostly of historical interest and should not be used:
int foo(void)
{
auto int i = 3;
int j = 5;
return 0;
}
2. register
Hints to the compiler that access to an object should be as fast as possible. The register storage class is more appropriate for variables that are defined inside a block and are accessed with high frequency. For example,
{
register int k, sum;
for(k = 1, sum = 0; k < 6; sum += k, k++);
printf("\t%d\n",sum);
}
In C11, The _Alignof
operator is also allowed to be used with register arrays.
3. extern
Used to declare an object or function that is defined elsewhere (and that has external linkage). In general, it is used to declare an object or function to be used in a module that is not the one in which the corresponding object or function is defined:
int foo = 2;
#include <stdio.h>
int main(void)
{
extern int foo;
printf("%d\n", foo);
return 0;
}
Things get slightly more interesting with the introduction of the inline keyword in C99:
Hints to the compiler that the function bar
might be inlined and suppresses the generation of an external symbol, unless stated otherwise.
inline void bar(int drink)
{
printf("You ordered drink no.%d\n", drink);
}
To be found in just one .c file. Creates an external function definition of bar
for use by other files. The compiler is allowed to choose between the inline version and the external definition when bar
is called. Without this line, bar
would only be an inline function, and other files would not be able to call it.
extern void bar(int);
4. static
The static storage class serves different purposes, depending on the location of the declaration in the file:
To confine the identifier to that translation unit only (scope=file).
static int i;
static int f(int n);
To save data for use with the next call of a function (scope=block):
void foo() {
static int a = 0;
int b = 0;
a += 10;
b += 10;
printf("static int a = %d, int b = %d\n", a, b);
}
int main(void) {
int i;
for (i = 0; i < 5; i++) {
foo();
}
return 0;
}
5. ___Thread_local
This was a new storage specifier introduced in C11 along with multi-threading. This isn't available in earlier C standards.
Denotes thread storage duration. A variable declared with _Thread_local
storage specifier denotes that the object is local to that thread and its lifetime is the entire execution of the thread in which it's created. It can also appear along with static or extern.
#include <threads.h>
#include <stdio.h>
#define SIZE 5
int thread_func(void *id)
{
static _Thread_local int i;
printf("From thread:[%d], Address of i (thread local): %p\n", *(int*)id, (void*)&i);
return 0;
}
int main(void)
{
thrd_t id[SIZE];
int arr[SIZE] = {1, 2, 3, 4, 5};
for(int i = 0; i < SIZE; i++) {
thrd_create(&id[i], thread_func, &arr[i]);
}
for(int i = 0; i < SIZE; i++) {
thrd_join(id[i], NULL);
}
}
6. typedef
Defines a new type based on an existing type. Its syntax mirrors that of a variable declaration.
typedef unsigned char Byte;
typedef int Integer[1];
typedef struct node *NodeRef;
typedef void (*SigHandler)(int);
While not technically a storage class, a compiler will treat it as one since none of the other storage classes are allowed if the typedef
keyword is used.
The typedefs are important and should not be substituted with #define macro
.
typedef int newType;
newType *ptr;
However,
#define int newType
newType *ptr;
Preprocessor Directives - C Programming
The C preprocessor is a macro processor that is used automatically by the C compiler to transform your program before actual compilation (Proprocessor direcives are executed before compilation.). It is called a macro processor because it allows you to define macros, which are brief abbreviations for longer constructs. A macro is a segment of code which is replaced by the value of macro. Macro is defined by #define
directive.
Preprocessing directives are lines in your program that start with #
. The #
is followed by an identifier that is the directive name. For example, #define
is the directive that defines a macro. Whitespace is also allowed before and after the #
.
The #
and the directive name cannot come from a macro expansion. For example, if foo
is defined as a macro expanding to define
, that does not make #foo
a valid preprocessing directive.
All preprocessor directives starts with hash # symbol.
List of preprocessor directives :
#include
#define
#undef
#ifdef
#ifndef
#if
#else
#elif
#endif
#error
#pragma
1. #include
The #include preprocessor directive is used to paste code of given file into current file. It is used include system-defined and user-defined header files. If included file is not found, compiler renders error. It has three variants:
#include <file>
This variant is used for system header files. It searches for a file named file in a list of directories specified by you, then in a standard list of system directories.
#include "file"
This variant is used for header files of your own program. It searches for a file named file first in the current directory, then in the same directories used for system header files. The current directory is the directory of the current input file.
#include anything else
This variant is called a computed #include
. Any #include
directive whose argument does not fit the above two forms is a computed include.
2. Macro's (#define)
Let's start with macro, as we discuss, a macro is a segment of code which is replaced by the value of macro. Macro is defined by #define
directive.
Syntax
#define token value
There are two types of macros:
- Object-like Macros
- Function-like Macros
1. Object-like Macros
The object-like macro is an identifier that is replaced by value. It is widely used to represent numeric constants. For example:
#define PI 3.1415
Here, PI is the macro name which will be replaced by the value 3.14. Let's see an example of Object-like Macros :
#include <stdio.h>
#define PI 3.1415
main()
{
printf("%f",PI);
}
Output:
3.14000
2. Function-like Macros
The function-like macro looks like function call. For example:
#define MIN(a,b) ((a)<(b)?(a):(b))
Here, MIN is the macro name. Let's see an example of Function-like Macros :
#include <stdio.h>
#define MIN(a,b) ((a)<(b)?(a):(b))
void main() {
printf("Minimum between 10 and 20 is: %d\n", MIN(10,20));
}
Output:
Minimum between 10 and 20 is: 10
A preprocessing directive cannot be more than one line in normal circumstances. It may be split cosmetically with Backslash-Newline. Comments containing Newlines can also divide the directive into multiple lines.
for example, you can split a line cosmetically with Backslash-Newline anywhere:
# defi\
ne FO\
O 10\
20
is equivalent into #define FOO 1020
.
3. #undef
To undefine a macro means to cancel its definition. This is done with the #undef
directive.
Syntax:
#undef token
define and undefine example
#include <stdio.h>
#define PI 3.1415
#undef PI
main() {
printf("%f",PI);
}
Output:
Compile Time Error: 'PI' undeclared
4. #ifdef
The #ifdef
preprocessor directive checks if macro is defined by #define
. If yes, it executes the code.
Syntax:
#ifdef MACRO
#endif
5. #ifndef
The #ifndef
preprocessor directive checks if macro is not defined by #define
. If yes, it executes the code.
Syntax:
#ifndef MACRO
#endif
6. #if
The #if
preprocessor directive evaluates the expression or condition. If condition is true, it executes the code.
Syntax:
#if expression
#endif
7. #else
The #else
preprocessor directive evaluates the expression or condition if condition of #if
is false. It can be used with #if
, #elif
, #ifdef
and #ifndef
directives.
Syntax:
#if expression
#else
#endif
Syntax with #elif
#if expression
#elif expression
#else
#endif
Example
#include <stdio.h>
#include <conio.h>
#define NUMBER 1
void main() {
#if NUMBER==0
printf("Value of Number is: %d",NUMBER);
#else
print("Value of Number is non-zero");
#endif
getch();
}
Output
Value of Number is non-zero
8. #error
The #error
preprocessor directive indicates error. The compiler gives fatal error if #error
directive is found and skips further compilation process.
C #error example
#include<stdio.h>
#ifndef __MATH_H
#error First include then compile
#else
void main(){
float a;
a=sqrt(7);
printf("%f",a);
}
#endif
9. #pragma
The #pragma
preprocessor directive is used to provide additional information to the compiler. The #pragma
directive is used by the compiler to offer machine or operating-system feature. Different compilers can provide different usage of #pragma directive.
Syntax:
#pragma token
Example
#include<stdio.h>
#include<conio.h>
void func() ;
#pragma startup func
#pragma exit func
void main(){
printf("\nI am in main");
getch();
}
void func(){
printf("\nI am in func");
getch();
}
Output
I am in func
I am in main
I am in func
Simple and Static Assertion (assert) in C Programming Language
An assertion is a statement used to assert that a fact must be true when that line of code is reached. Assertions are useful for ensuring that expected conditions are met.
1. Simple Assertion
Simple assertion can be implemented using assert(expression)
method of assert.h
header file.
Syntax
assert(expression)
Parameters
- expression - expression of scalar type
When the condition passed to an assertion is true, there is no action. The behaviour on false conditions depends on compiler flags. When assertions are enabled, a false input causes an immediate program halt. When they are disabled, no action is taken. It is common practice to enable assertions in internal and debug builds, and disable them in release builds, though assertions are often enabled in release (Whether termination is better or worse than errors depends on the program.)
Assertions should be used only to catch internal programming errors, which usually means being passed bad parameters.
Example
We are going to use gcc
compiler on Windows 10 using Bash on Ubuntu on Windows. You can use any other compiler but If you've Windows 10 pc you can install gcc
compiler on Windows 10. Read this post to learn how to install gcc compiler on Windows 10 using Bash on Ubuntu on Windows
#include <stdio.h>
#include <assert.h>
int main(void)
{
int x;
printf("Enter the value of x");
scanf("%d",&x);
assert(x >= 0);
printf("x = %d\n", x);
return 0;
}
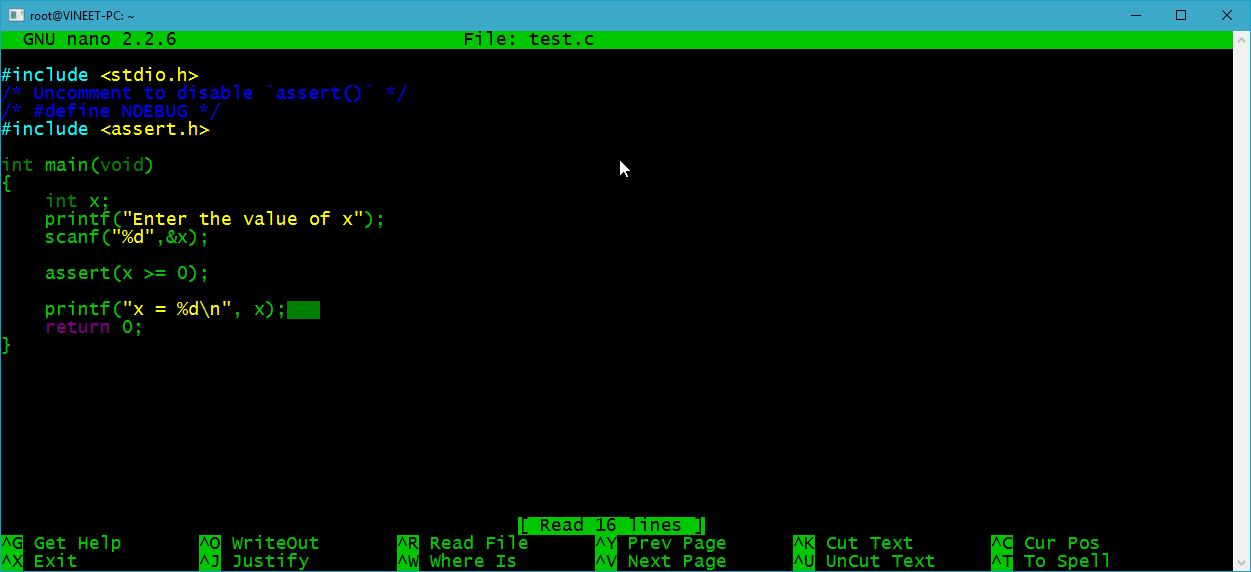
Possible output for x = -1 with NDEBUG undefined:
a.out: main.c:12: main: Assertion `x >= 0' failed.
Possible output for x = -1 with NDEBUG defined:
x = -1
Here the more test cases
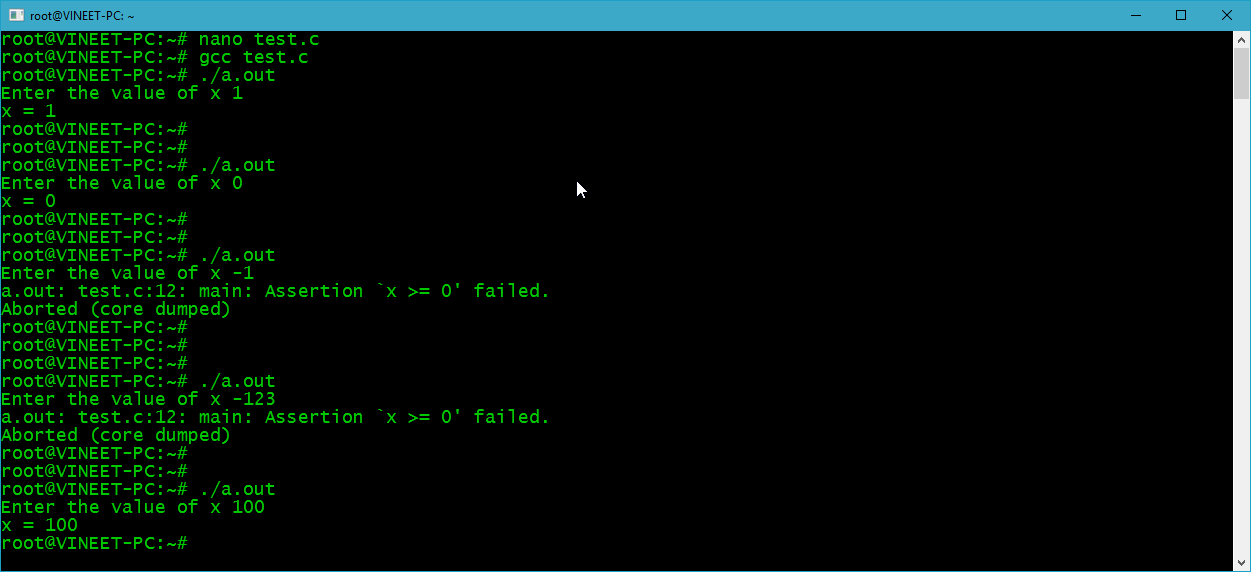
2. Static Assertions (C11)
Static assertions are used to check if a condition is true when the code is compiled. If it isn't, the compiler is required to issue an error message and stop the compiling process.
A static assertion is one that is checked at compile time, not run time. The condition must be a constant expression, and if false will result in a compiler error. The first argument, the condition that is checked, must be a constant expression, and the second a string literal.
Unlike assert, _Static_assert
is a keyword. A convenience macro static_assert
is also defined in assert.h
header file. Static assertion only available in C11 version of C..
Syntax
static_assert(expression, message)
"or"
_Static_assert(expression, message)
Parameters
- expression - expression of scalar type.
- message - string literal to be included in the diagnostic message.
Example
#include <assert.h>
enum
{
N = 5
};
_Static_assert(N == 5, "N does not equal 5");
static_assert(N > 10, "N is not greater than 10");
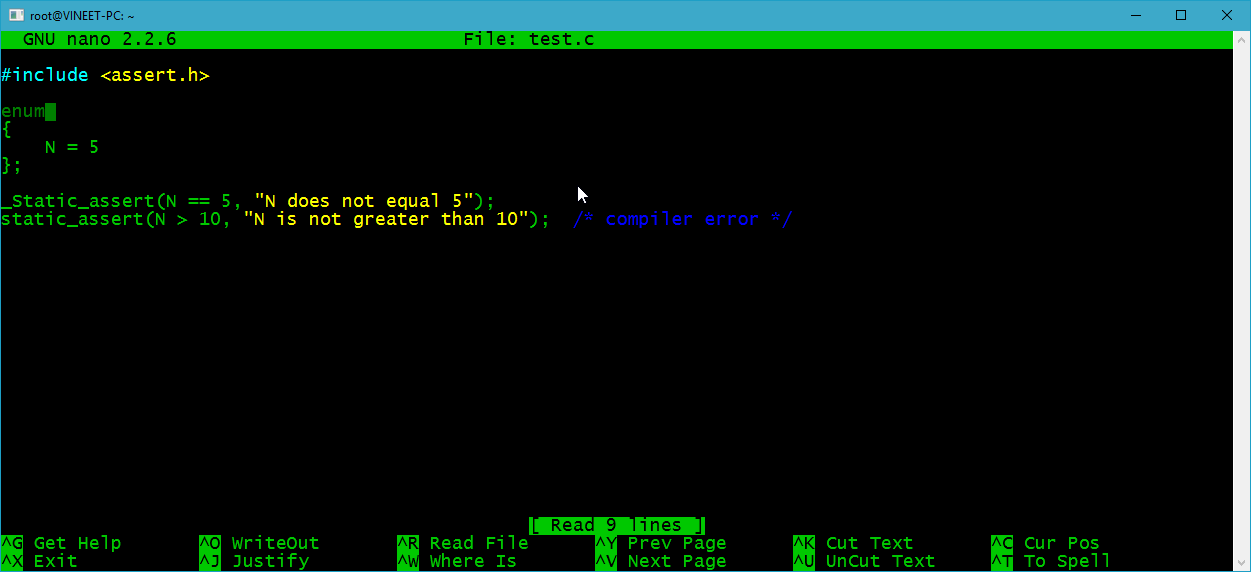
Possible output:
test.c:9:17: error: expected ‘)’ before ‘>’ token
static_assert(N > 10, "N is not greater than 10"); /* compiler error */
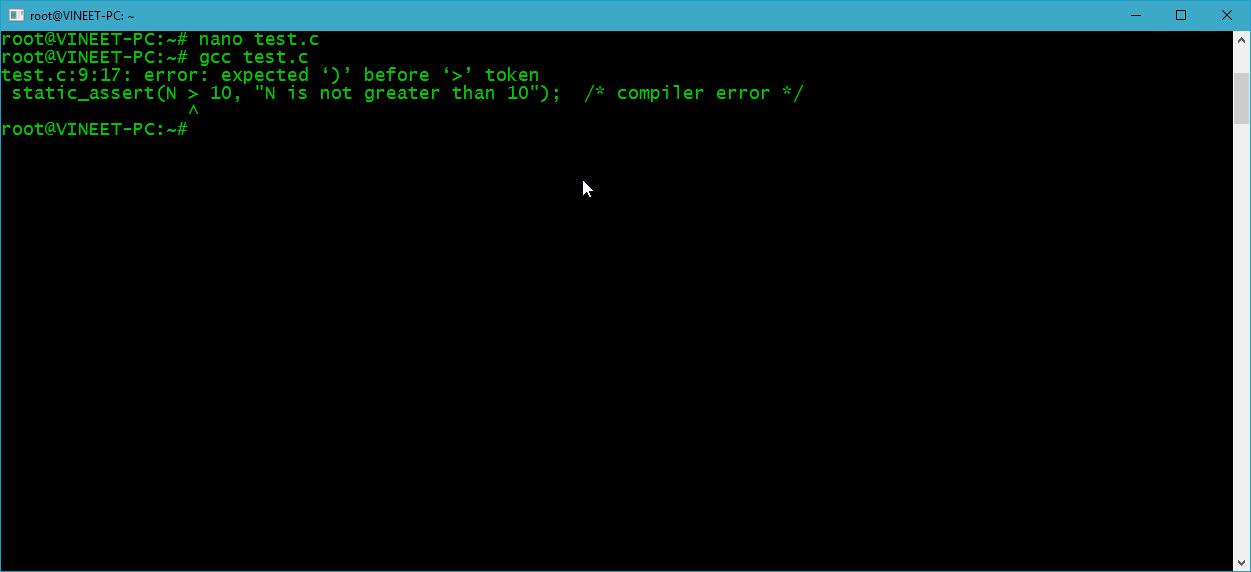
3. Trick to display error message in Simple Assertion
A trick exists that can display an error message along with an assertion. Normally, you would write code like this
void f(void *p)
{
assert(p != NULL);
}
If the assertion failed, an error message would resemble
Assertion failed: p != NULL, file main.c, line 5
However, you can use logical AND
(&&
) to give an error message as well
void f(void *p)
{
assert(p != NULL && "function f: p cannot be NULL");
}
Now, if the assertion fails, an error message will read something like this
Assertion failed: p != NULL && "function f: p cannot be NULL", file main.c, line 5
The reason as to why this works is that a string literal always evaluates to non-zero (true
). Adding &&
1 to a Boolean expression has no effect. Thus, adding &&
"error message" has no effect either, except that the compiler will display the entire expression that failed.
Graphics (graphics.h) - C Programming
Graphics programming in C used to drawing various geometrical shapes(rectangle, circle eclipse etc), use of mathematical function in drawing curves, coloring an object with different colors and patterns and simple animation programs like jumping ball and moving cars.
1. First graphics program (Draw a line)
#include<graphics.h>
#include<stdio.h>
#include<conio.h>
void main(void) {
int gdriver = DETECT, gmode;
int x1 = 200, y1 = 200;
int x2 = 300, y2 = 300;
clrscr();
initgraph(&gdriver, &gmode, "c:\\turboc3\\bgi");
line(x1, y1, x2, y2);
getch();
closegraph();
}
2. Explanation of Code :
The first step in any graphics program is to include graphics.h
header file. The graphics.h
header file provides access to a simple graphics library that makes it possible to draw lines, rectangles, ovals, arcs, polygons, images, and strings on a graphical window.
The second step is initialize the graphics drivers on the computer using initgraph
method of graphics.h
library.
void initgraph(int *graphicsDriver, int *graphicsMode, char *driverDirectoryPath);
It initializes the graphics system by loading the passed graphics driver then changing the system into graphics mode. It also resets or initializes all graphics settings like color, palette, current position etc, to their default values. Below is the description of input parameters of initgraph function.
-
graphicsDriver : It is a pointer to an integer specifying the graphics driver to be used. It tells the compiler that what graphics driver to use or to automatically detect the drive. In all our programs we will use DETECT
macro of graphics.h library that instruct compiler for auto detection of graphics driver.
-
graphicsMode : It is a pointer to an integer that specifies the graphics mode to be used. If *gdriver
is set to DETECT
, then initgraph
sets *gmode
to the highest resolution available for the detected driver.
-
driverDirectoryPath : It specifies the directory path where graphics driver files (BGI files
) are located. If directory path is not provided, then it will search for driver files in current working directory directory. In all our sample graphics programs, you have to change path of BGI directory accordingly where you Turbo C++ compiler is installed.
We have declared variables so that we can keep track of starting and ending point.
int x1=200, y1=200;
int x2=300, y2=300;
No, We need to pass just 4 parameters to the line
function.
line(x1,y1,x2,y2);
line
Function Draws Line From (x1,y1) to (x2,y2) .
Syntax : line(x1,y1,x2,y2);
Parameter Explanation
- x1 - X Co-ordinate of First Point
- y1 - Y Co-ordinate of First Point
- x2 - X Co-ordinate of Second Point
- y2 - Y Co-ordinate of Second Point
At the end of our graphics program, we have to unloads the graphics drivers and sets the screen back to text mode by calling closegraph
function.
3. Colors in C Graphics Programming
There are 16 colors declared in graphics.h header file. We use colors to set the current drawing color, change the color of background, change the color of text, to color a closed shape etc (Foreground and Background Color). To specify a color, we can either use color constants like setcolor(RED), or their corresponding integer codes like setcolor(4). Below is the color code in increasing order.
CONSTANT | VALUE | BACKGROUND? | FOREGROUND? |
BLACK |
0 |
Yes |
Yes |
BLUE |
1 |
Yes |
Yes |
GREEN |
2 |
Yes |
Yes |
CYAN |
3 |
Yes |
Yes |
RED |
4 |
Yes |
Yes |
MAGENTA |
5 |
Yes |
Yes |
BROWN |
6 |
Yes |
Yes |
LIGHTGRAY |
7 |
Yes |
Yes |
DARKGRAY |
8 |
NO |
Yes |
LIGHTBLUE |
9 |
NO |
Yes |
LIGHTGREEN |
10 |
NO |
Yes |
LIGHTCYAN |
11 |
NO |
Yes |
LIGHTRED |
12 |
NO |
Yes |
LIGHTMAGENTA |
13 |
NO |
Yes |
YELLOW |
14 |
NO |
Yes |
WHITE |
15 |
NO |
Yes |
BLINK |
128 |
NO |
* |
***** To display blinking characters in text mode, add BLINK to the foreground color. (Defined in conio.h
)
4. Graphics example using color
#include<graphics.h>
#include<stdio.h>
#include<conio.h>
void main()
{
int gd = DETECT, gm;
clrscr();
initgraph(&gd, &gm, "C:\\TURBOC3\\BGI");
setcolor(BLUE);
rectangle(50,50,100,100);
getch();
closegraph();
}
5. Examples
EXAMPLE STATEMENT FOR GRAPHICS IN C LANGUAGE |
1. Drawing Line in Graphics Mode
|
#include<graphics.h>
#include<stdio.h>
#include<conio.h>
void main(void) {
int gdriver = DETECT, gmode;
int x1 = 200, y1 = 200;
int x2 = 300, y2 = 300;
clrscr();
initgraph(&gdriver, &gmode, "c:\\turboc3\\bgi");
line(x1, y1, x2, y2);
closegraph();
getch();
}
2. Make Static Countdown
|
#include <graphics.h>
#include <dos.h>
#include <conio.h>
int main()
{
int gd = DETECT, gm, i;
char a[5];
initgraph( &gd, &gm, "C:\\TURBOC3\\BGI");
settextjustify( CENTER_TEXT, CENTER_TEXT );
settextstyle(DEFAULT_FONT,HORIZ_DIR,50);
setcolor(RED);
for (i = 30; i >=0; i--)
{
sprintf(a,"%d",i);
outtextxy(getmaxx()/2, getmaxy()/2, a);
delay(1000);
if ( i == 0 )
break;
cleardevice();
}
cleardevice();
settextstyle(SANS_SERIF_FONT,HORIZ_DIR,2);
outtextxy(300,300,"Project by http://www.turboc.codeplex.com");
delay(5000);
closegraph();
return 0;
}
3. Draw Moving a Car
|
#include <graphics.h>
#include <dos.h>
#include <conio.h>
main()
{
int i, j = 0, gd = DETECT, gm;
initgraph(&gd,&gm,"C:\\TURBOC3\\BGI");
settextstyle(DEFAULT_FONT,HORIZ_DIR,2);
outtextxy(25,240,"Press any key to view the moving car");
getch();
setviewport(0,0,639,440,1);
for( i = 0 ; i <= 420 ; i = i + 10, j++ )
{
rectangle(50+i,275,150+i,400);
rectangle(150+i,350,200+i,400);
circle(75+i,410,10);
circle(175+i,410,10);
setcolor(j);
delay(100);
if( i == 420 )
break;
clearviewport();
}
getch();
cleardevice();
settextstyle(SANS_SERIF_FONT,HORIZ_DIR,2);
outtextxy(100,200,"Project by http://www.turboc.codeplex.com");
delay(5000);
closegraph();
return 0;
}
4. Press Me Button Game
|
#include <stdio.h>
#include <conio.h>
#include <dos.h>
#include <graphics.h>
#include <stdlib.h>
union REGS i, o;
int left = 265, top = 250;
void initialize_graphics_mode()
{
int gd = DETECT, gm, error;
initgraph(&gd,&gm,"C:\\TURBOC3\\BGI");
error = graphresult();
if (error != grOk)
{
perror("Error ");
printf("Press any key to exit...\n");
getch();
exit(EXIT_FAILURE);
}
}
void showmouseptr()
{
i.x.ax = 1;
int86(0x33,&i,&o);
}
void hidemouseptr()
{
i.x.ax = 2;
int86(0x33,&i,&o);
}
void getmousepos(int *x,int *y)
{
i.x.ax = 3;
int86(0x33,&i,&o);
*x = o.x.cx;
*y = o.x.dx;
}
void draw_bar()
{
hidemouseptr();
setfillstyle(SOLID_FILL,CYAN);
bar(190,180,450,350);
showmouseptr();
}
void draw_button(int x, int y)
{
hidemouseptr();
setfillstyle(SOLID_FILL,MAGENTA);
bar(x,y,x+100,y+30);
moveto(x+5,y);
setcolor(YELLOW);
outtext("Press me");
showmouseptr();
}
void draw()
{
settextstyle(SANS_SERIF_FONT,HORIZ_DIR,2);
outtextxy(100,451,"Project by http://www.turboc.codeplex.com");
setcolor(BLUE);
rectangle(0,0,639,450);
setcolor(RED);
outtextxy(160,25,"Try to press the \"Press me\" button");
outtextxy(210,50,"Press escape key to exit");
setfillstyle(XHATCH_FILL,GREEN);
setcolor(BLUE);
bar(1,1,75,449);
bar(565,1,638,449);
showmouseptr();
draw_bar();
draw_button(left,top);
}
void initialize()
{
initialize_graphics_mode();
if( !initmouse() )
{
closegraph();
printf("Unable to initialize the mouse");
printf("Press any key to exit...\n");
getch();
exit(EXIT_SUCCESS);
}
draw();
}
int initmouse()
{
i.x.ax = 0;
int86(0x33,&i,&o);
return ( o.x.ax );
}
void get_input()
{
int x, y;
while(1)
{
getmousepos(&x,&y);
if( x >= (left-3) && y >= (top-3) && y <= (top+30+3) && x < left )
{
draw_bar();
left = left + 4;
if (left > 350)
left = 190;
draw_button(left,top);
}
else if (x<=(left+100+3)&&y>=(top-3)&&y<=(top+30+3)&&x>(left+100))
{
draw_bar();
left = left - 4;
if (left < 190)
left = 350;
draw_button(left,top);
}
else if(x>(left-3) && y>=(top-3) && y<(top) && x<= (left+100+3))
{
draw_bar();
top = top + 4;
if (top > 320)
top = 180;
draw_button(left,top);
}
else if (x>(left-3)&&y>(top+30)&&y<=(top+30+3)&&x<=(left+100+3))
{
draw_bar();
top = top - 4;
if (top < 180)
top = 320;
draw_button(left,top);
}
if (kbhit())
{
if (getkey() == 1)
exit(EXIT_SUCCESS);
}
}
}
int getkey()
{
i.h.ah = 0;
int86(22,&i,&o);
return( o.h.ah );
}
main()
{
initialize();
get_input();
return 0;
}
5. Draw Smiling Face Animation
|
#include<graphics.h>
#include<conio.h>
#include<stdlib.h>
main()
{
int gd = DETECT, gm, area, temp1, temp2, left = 25, top = 75;
void *p;
initgraph(&gd,&gm,"C:\\TURBOC3\\BGI");
setcolor(YELLOW);
circle(50,100,25);
setfillstyle(SOLID_FILL,YELLOW);
floodfill(50,100,YELLOW);
setcolor(BLACK);
setfillstyle(SOLID_FILL,BLACK);
fillellipse(44,85,2,6);
fillellipse(56,85,2,6);
ellipse(50,100,205,335,20,9);
ellipse(50,100,205,335,20,10);
ellipse(50,100,205,335,20,11);
area = imagesize(left, top, left + 50, top + 50);
p = malloc(area);
setcolor(WHITE);
settextstyle(SANS_SERIF_FONT,HORIZ_DIR,2);
outtextxy(20,451,"Smiling Face Project by http://www.turboc.codeplex.com");
setcolor(BLUE);
rectangle(0,0,639,449);
while(!kbhit())
{
temp1 = 1 + random ( 588 );
temp2 = 1 + random ( 380 );
getimage(left, top, left + 50, top + 50, p);
putimage(left, top, p, XOR_PUT);
putimage(temp1 , temp2, p, XOR_PUT);
delay(500);
left = temp1;
top = temp2;
}
getch();
closegraph();
return 0;
}
6. Make Traffic Light Simulation
|
#include<graphics.h>
#include<conio.h>
#include<dos.h>
#include<stdlib.h>
main()
{
int gd = DETECT, gm, midx, midy;
initgraph(&gd, &gm, "C:\\TURBOC3\\BGI");
midx = getmaxx()/2;
midy = getmaxy()/2;
setcolor(RED);
settextstyle(SCRIPT_FONT, HORIZ_DIR, 3);
settextjustify(CENTER_TEXT, CENTER_TEXT);
outtextxy(midx, midy-10, "Traffic Light Simulation");
outtextxy(midx, midy+10, "Press any key to start");
getch();
cleardevice();
setcolor(WHITE);
settextstyle(DEFAULT_FONT, HORIZ_DIR, 1);
rectangle(midx-30,midy-80,midx+30,midy+80);
circle(midx, midy-50, 22);
setfillstyle(SOLID_FILL,RED);
floodfill(midx, midy-50,WHITE);
setcolor(BLUE);
outtextxy(midx,midy-50,"STOP");
delay(2000);
graphdefaults();
cleardevice();
setcolor(WHITE);
rectangle(midx-30,midy-80,midx+30,midy+80);
circle(midx, midy, 20);
setfillstyle(SOLID_FILL,YELLOW);
floodfill(midx, midy,WHITE);
setcolor(BLUE);
outtextxy(midx-18,midy-3,"READY");
delay(2000);
cleardevice();
setcolor(WHITE);
rectangle(midx-30,midy-80,midx+30,midy+80);
circle(midx, midy+50, 22);
setfillstyle(SOLID_FILL,GREEN);
floodfill(midx, midy+50,WHITE);
setcolor(BLUE);
outtextxy(midx-7,midy+48,"GO");
setcolor(RED);
settextstyle(SCRIPT_FONT, HORIZ_DIR, 4);
outtextxy(midx-150, midy+100, "Press any key to exit...");
getch();
clrscr();
cleardevice();
setcolor(RED);
settextstyle(SCRIPT_FONT, HORIZ_DIR, 3);
settextjustify(CENTER_TEXT, CENTER_TEXT);
outtextxy(midx, midy, "Project by http://www.turboc.codeplex.com");
delay(5000);
closegraph();
return 0;
}