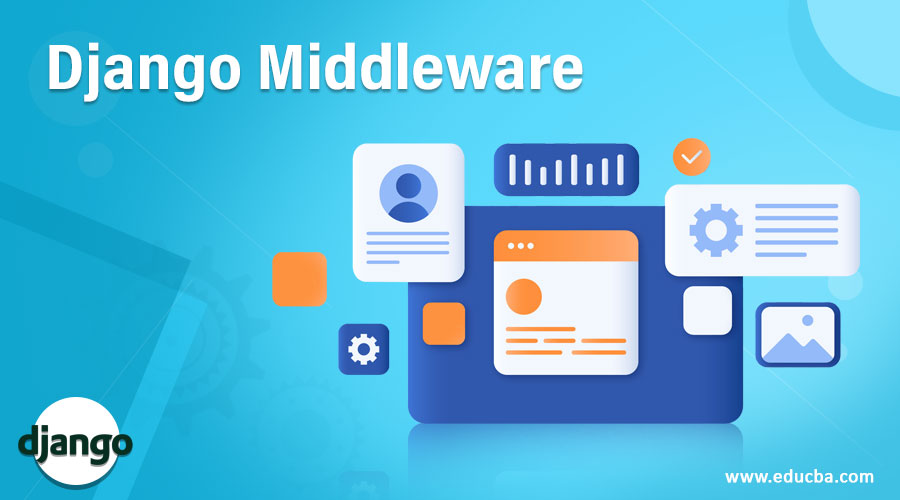
Introduction to Django Middleware
The middleware has to pull every request and response to the web application and process the middleware’s processing over request and response. On a simple, these middleware components are nothing more than a Python class; these middleware elements are called components, each of these components is responsible for performing some operation. These components are represented as the strings in the Python list variable MIDDLEWARE in the settings.py file. The middleware components can be declared in any of the paths in the application; the only thing to be ensured is whether that path is declared in the Middleware tuple in the settings.py file. In this topic, we are going to learn about Django Middleware.
Middleware Activation
A middleware can be activated by mentioning the middleware item in the MIDDLEWARE list in the settings.py file. The list below holds the default list of middleware items generated when a Django project starts. The order in which the middleware components are declared is significant.
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
The necessity and usage of the above-listed default middlewares in the Django framework are explained below,
Default Middlewares |
Operations |
Descriptions |
djangosecure. Middleware.SecurityMiddleware |
X-Frame-Options: DENY |
Limits the pages displayed to be within a frame. |
HTTP Strict Transport Security |
Setting this allows the website to be accessible to the browser only on HTTPS instead of HTTP. |
X-Content-Type-Options: nosniff |
This option helps to prevent against MIME sniffing vulnerabilities. |
X-XSS-Protection: 1; mode=block |
When an XSS(Cross-site scripting) attack is detected, it stops the website pages from getting further loaded. |
SSL Redirect |
This will redirect all the http connections to https connections. |
Detecting proxied SSL |
At rare instances, the request.is_secure(), the method returns false for valid requests; setting this option will help to set an alternative header to the secured external connection. |
Django. middleware.common.CommonMiddleware |
Rewriting of URLs based on APPEND_SLASH and PREPEND_WWW settings. When APPEND_SLASH is TRUE, and the URL does not have a ‘/,’ then the new URL will have a slash at the end of the URL. |
NA |
Restrict access for the users listed in DISALLOWED_USER_AGENTS setting |
django.contrib.sessions. middleware.SessionMiddleware |
When the session Middleware is activated, every HttpRequest object will have a session attribute tagged as the first argument. This is a dictionary kind of object, and session values can be inserted into this dictionary object using a request. Session anywhere in the view file. |
Django. middleware.csrf.CsrfViewMiddleware |
This middleware option allows protection against all cross-site request forgeries. |
django.contrib.auth. middleware.AuthenticationMiddleware |
Setting this middleware option adds a currently logged-in user to every httpRequest object which is entered in. |
django.contrib.messages. middleware.MessageMiddleware |
This middleware will handle all temporary messages between the website and the web browser. |
django. middleware.clickjacking.XFrameOptionsMiddleware |
Limits the pages displayed to be within a frame. |
How does middleware work in Django?
Below are the key points on the working of middleware in Django,
- The order in which the middleware components are declared is significant.
- The middleware classes get executed twice in the request/response lifecycle.
- During a request, the classes are executed from top to bottom order.
- During a response, these classes get executed from bottom to top order. This is why the order of the components is significant.
- The _init_ method is executed during the start of the server.
- the _call__ method is executed for every request.
Mandatory Methods in a Middleware
At least one among the below-listed methods must be declared as a part of the middleware,
- If middleware needs to process during request:
- process_request(request)
- process_view(request, view_func, view_args, view_kwargs)
- If middleware needs to process during response:
- process_exception(request, exception) (only if the view raised an exception)
- process_template_response(request, response) (only for template responses)
- process_response(request, response)
Custom Middleware
For setting up a custom middleware setup in place, the below-mentioned steps are expected to be followed; This custom middleware will be a user-defined middleware staying in the middle and doing the needed or guided set of processing on the passed request and response messages. Custom middleware offers great flexibility to middleware services. So these custom-level middleware services play a very important role in Django middleware setups. Additionally, the properties of built-in middleware services can also be modulated to several possible extents to attain middleware capabilities.
1. Place a file called middleware.py anywhere inside the project. The location at which this middleware file has been placed is not a big deal as per the Django setup. What matters is the process of ensuring the path placed for this file is precisely mentioned in the middleware list in the settings.py file. This is the most important element.
2. Place the class for the middleware inside the middleware.py file. This is the most important step in this process; Since the order in which the middleware gets executed is a great deal, it is important to ensure that the newly added middleware item is placed as the last element in the middleware listing. Also, it needs to be strictly ensured that the middleware item is of string format,
middleware.py:
class NewMiddleware:
def __init__(self, get_request):
self.get_request = get_request
def __call__(self, request):
request = self.get_request(request)
print("The Newly installed middleware is successfully triggered!!!")
return request
MIDDLEWARE =
[
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
'Django_app1.middleware.NewMiddleware',
]
From the above-mentioned entry, it could be decided that the middleware.py file is placed inside the Django_app1 folder, and the file has a middleware class named NewMiddleware inside it as an instance. If several more new middlewares have to be installed in the middleware.py file, then separate entries for all those have to be placed.
3. Reload the server using the python manage.py runserver command and verify the webpage.
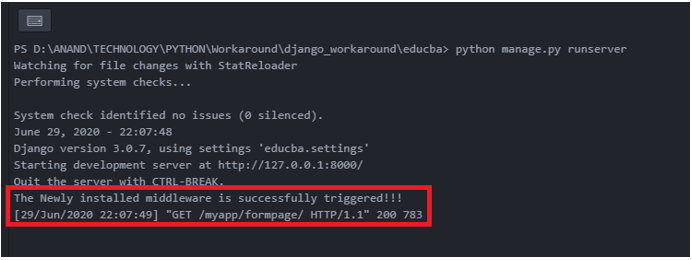
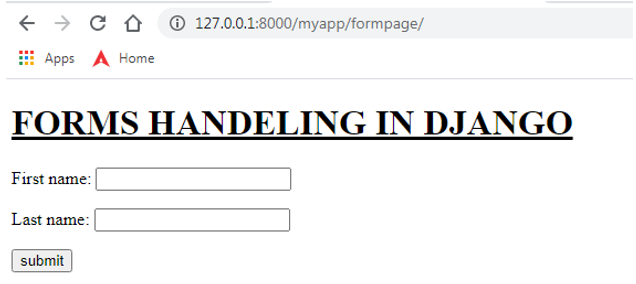
Once the server is loaded and any request is received from the page, the middle message mentioning “The Newly installed middleware is successfully triggered!!!” is displayed on the screen. This proves the newly installed middleware is working as expected.
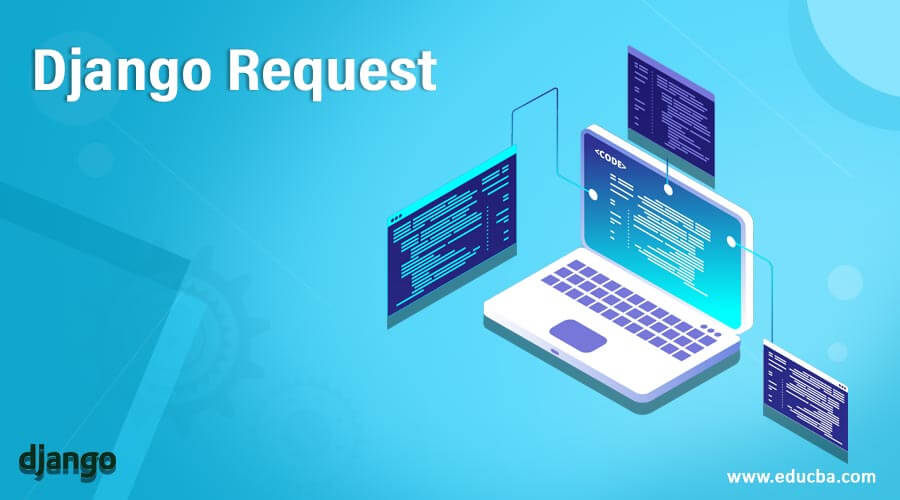
Introduction to Django Request
Web API Requests are a part of the REST framework structure. The REST frameworks expand as Representational State Transfer. These REST frameworks are responsible for web API data communication. The REQUEST is a component of the REST framework structure. The REQUEST is responsible for passing a request into the web API system; based on the Request posted, the API system makes processing and delivers the response. Based on the request being brought into the web API system, the system will calculate and provide the necessary output. The technique through which the request api’s are formulated in the Django setup is very optimized and stable structuring.
HTTP Methods
The various methods used in the Django request.method are listed below,
HTTP Methods |
Description |
GET |
The GET method requests a specified resource and retrieves the data. The response for GET will have a HEAD and BODY. |
PUT |
The PUT method is used for updating the specified resource. |
POST |
The POST method is used for submitting an entity to a specified resource. |
HEAD |
The HEAD method is very similar to GET, but the response for a HEAD doesn’t have a body segment. |
DELETE |
The DELETE method is used for deleting a resource. |
CONNECT |
The CONNECT method establishes a connection between the Target and the source. |
OPTIONS |
Mentions all the options between Target and source |
TRACE |
It depicts the path between Target and Source |
PATCH |
Any partial modifications are applied to the PATH resource. |
Request Framework Attributes
The attributes of the Django request framework are listed below,
Django Request attributes |
Description |
HttpRequest.scheme |
The request scheme is represented as a string. |
HttpRequest.body |
A byte string representation of the request body |
HttpRequest.path |
The entire path of the requested page is printed |
HttpRequest.path_info |
This mentions the path info portion of the path |
HttpRequest.method |
Denotes the type of HTTP request triggered, e.g., GET, POST, etc |
HttpRequest.encoding |
Mentions the type of encoding used with the request; if the encoding is not specified, it is mentioned as None. |
HttpRequest.content_type |
This Denotes the MIME type of the request, parsed from the CONTENT_TYPE header. |
HttpRequest.content_params |
All the key-value parameters mentioned in the Content-type Header will be denoted here. |
HttpRequest.GET |
Returns the parameter for GET |
HttpRequest.POST |
Returns the parameter for POST |
HttpRequest.COOKIES |
All COOKIES details are denoted here |
HttpRequest.FILES |
It contains all the uploaded files. |
HttpRequest.META |
All HTTP headers are denoted in the META attribute. |
HttpRequest.resolver_match |
It contains an instance of ResolverMatch representing the resolved URL. |
Request Framework Methods
All methods associated with the request framework are mentioned below,
Django Request methods |
Description |
HttpRequest.get_host() |
Returns the current host details. |
HttpRequest.get_port() |
Returns the currently connected port details. |
HttpRequest.get_full_path() |
Returns the entire path. |
HttpRequest.build_absolute_uri (location) |
It returns the absolute URI form of a location. |
HttpRequest.get_signed_cookie (key, default=RAISE_ERROR, salt=”, max_age=None) |
Assigns a cookie value for a signed cookie |
HttpRequest.is_secure() |
Denotes True if the connection is secure |
HttpRequest.is_ajax() |
Denotes True if the request is made via Ajax setup |
Examples of Django Request
Different examples are mentioned below:
Example #1
Design the choiceField() in the forms.py with the values it needs to display and process in the choices attribute of the field.
forms.py:
from django import forms
class requestcheckform(forms.Form):
request_attribute = forms.ChoiceField(choices=[('Request Header','Request Header'),
('Request POST','Request POST'),
('Request Files','Request Files'),
('Request GET','Request GET'),
('Request User','Request User'),
('Request Body','Request Body'),
('Request Content Type','Request Content Type'),
('Request Encoding','Request Encoding'),
('Request Method','Request Method'),
('Request Cookies','Request Cookies'),
('Request Path','Request Path'),
('Request META','Request META'),
('Request port','Request port'),
('Request host','Request host'),
('Request is_secure','Request is_secure'),
Example #2
Formulate the view function for capturing all the request attributes and methods and post them to the messages. Success () prompt allows us to prompt the messages retrieved again into the screen.
views.py:
from django.shortcuts import render
from django.http import HttpResponse
from Django_app1.forms import Valueform,fileform,emailform,requestcheckform
import requests
def requestmethods(request):
form = requestcheckform()
if request.method == 'POST':
if request.POST['request_attribute'] == 'Request Header':
data_content = "data content of request method: " + str(request.headers)
messages.success(request,data_content)
elif request.POST['request_attribute'] == 'Request POST':
post_content = "post content of request method: " + str(request.POST)
messages.success(request,post_content)
elif request.POST['request_attribute'] == 'Request Files':
FILES_content = "FILES in request method: " + str(request.FILES)
messages.success(request,FILES_content)
elif request.POST['request_attribute'] == 'Request GET':
GET_content = "GET Content in request method: " + str(request.GET)
messages.success(request,GET_content)
elif request.POST['request_attribute'] == 'Request User':
Request_User = "User Details: " + str(request.user)
messages.success(request,Request_User)
elif request.POST['request_attribute'] == 'Request Body':
Request_body = "Request Body: " + str(request.body)
messages.success(request,Request_body)
elif request.POST['request_attribute'] == 'Request Content Type':
Request_Content_Type = "Request Content type: " + str(request.content_type)
messages.success(request,Request_Content_Type)
elif request.POST['request_attribute'] == 'Request Encoding':
Request_Encoding = "Request Encoding Used: " + str(request.encoding)
messages.success(request,Request_Encoding )
elif request.POST['request_attribute'] == 'Request Method':
Request_method = "Request Method posted: " + str(request.method)
messages.success(request,Request_method )
elif request.POST['request_attribute'] == 'Request Path':
Request_path = "Path of the request: " + str(request.path)
messages.success(request,Request_path )
elif request.POST['request_attribute'] == 'Request Cookies':
Request_Cookies = "Cookies associated to the Request: " + str(request.COOKIES)
messages.success(request,Request_Cookies )
elif request.POST['request_attribute'] == 'Request META':
Request_META = "HTTP headers info: " + str(request.META)
messages.success(request,Request_META )
elif request.POST['request_attribute'] == 'Request port':
Request_port = "Request port number: " + str(request.get_port())
messages.success(request,Request_port )
elif request.POST['request_attribute'] == 'Request host':
Request_host = "Requested Host: " + str(request.get_host())
messages.success(request,Request_host)
elif request.POST['request_attribute'] == 'Request is_secure':
Request_secure = "Security level of the request: " + str(request.is_secure())
messages.success(request,Request_secure)
return render(request,'Request_methods_check.html',{"form":form})
Example #3
Design the webpage in the corresponding template file for this page,
Request_methods_check.html:
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<title>Django App1</title>
{% load static %}
<link href="{% static 'admin/css/font.css' %}" rel="stylesheet">
<style>
body {
background-image: url("{% static 'admin/img/background1.jpeg' %}");
background-color: #acccbb;
}
</style>
</head>
<body>
<h1> <u> HTTP METHODS CHECK </u> </h1>
<form method='POST'>
{% csrf_token %}
<button type='submit' onclick="message()"> Click to check Head() and OPTIONS() response </button>
</form>
<form method='GET'>
{% csrf_token %}
<button type='submit' onclick="message()"> Click to check GET() response </button>
</form>
<ul class="messages">
{% for message in messages %}
<li{% if message.tags %} class="{{ message.tags }}"{% endif %}>{{ message }}</li>
{% endfor %}
</ul>
</body>
</html>
Output:
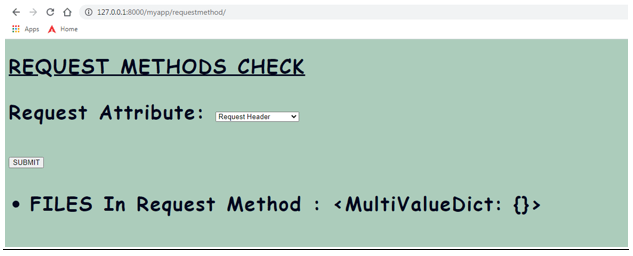
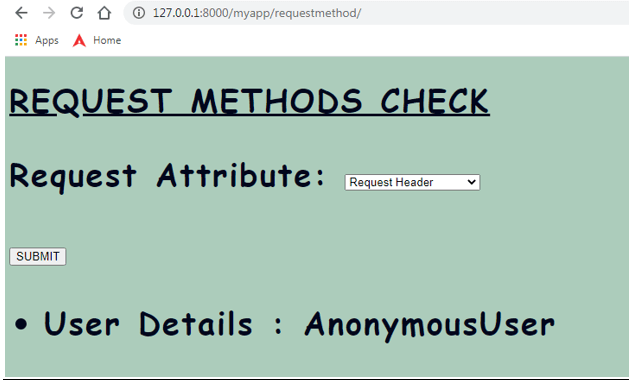
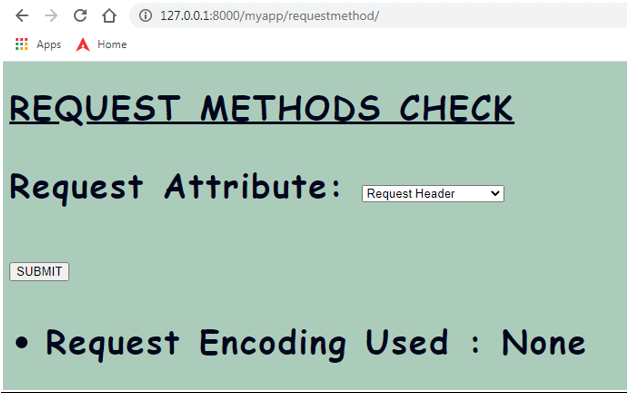
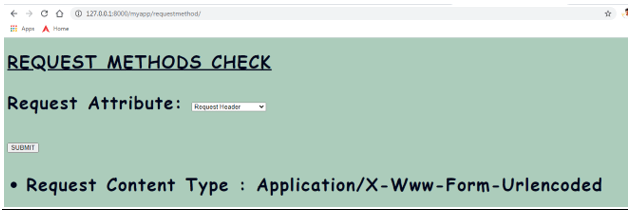
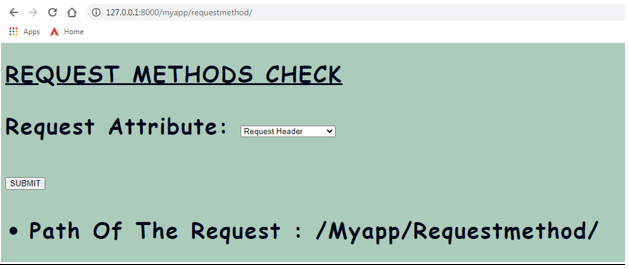
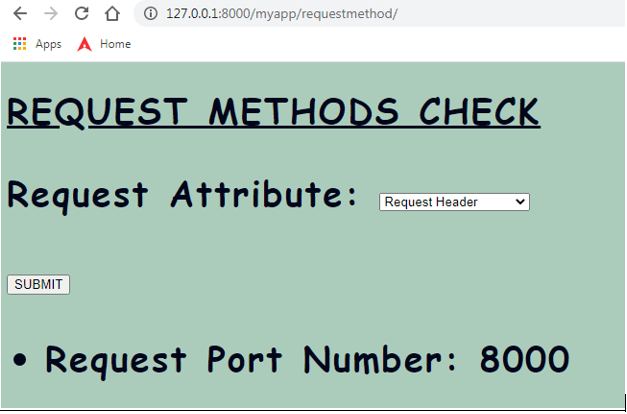
Conclusion – Django Request
All methods, attributes, and elements of the request API structure are briefly explained with suitable examples for every request item triggered.